.输入一个整数,若为奇数则输出其平方跟,否则输出其立方根。要求分别用单分支、双分支及条件运算实现。
时间: 2024-12-19 16:19:54 浏览: 3
当然可以,这里我会分别用单分支、双分支以及条件运算符的方式给你展示如何编写这个程序。
1. 单分支结构 (using if-else):
```python
num = int(input("请输入一个整数: "))
if num % 2 != 0: # 判断是否为奇数
result = num ** 2 # 如果是奇数,计算平方
else:
result = num ** (1/3) # 否则是奇数,计算立方根
print("结果:", result)
```
2. 双分支结构 (using if-elif-else):
```python
num = int(input("请输入一个整数: "))
if num % 2 == 0: # 判断是否为偶数
print(f"{num} 的立方根是 {num ** (1/3)}")
else:
print(f"{num} 的平方是 {num ** 2}")
```
3. 条件运算符 (using the ternary operator):
```python
num = int(input("请输入一个整数: "))
result = num ** (num % 2 * 2 - 1) # 根据num%2的结果决定是平方还是立方根
print(f"结果: {result}")
```
相关问题
python:输入一个整数,若为奇数则输出其平方跟,否则输出其立方根。要求分别用单分支、双分支及条件运算实现
在Python中,你可以使用if-elif-else结构(双分支)、单个if条件判断以及三元表达式(条件运算符)来实现这个功能。以下是三种方式的代码示例:
**双分支(if-elif-else)实现:**
```python
num = int(input("请输入一个整数:"))
result = None
if num % 2 == 1: # 单分支判断是否为奇数
result = num ** 2 # 平方
else:
result = num ** (1/3) # 立方根,使用幂运算表示
print(result)
```
**单分支(if)实现:**
```python
num = int(input("请输入一个整数:"))
result = num ** (2 if num % 2 == 1 else 1/3) # 使用嵌套的条件判断
print(result)
```
**条件运算符(三元表达式)实现:**
```python
num = int(input("请输入一个整数:"))
result = num ** (2 if num % 2 != 0 else 1/3) # 使用三元运算符简化代码
print(result)
```
输入一个整数,若为奇数则输出其平方根,否则输出其立方根。要求分别用单分支、双分支及条件运算实现java
单分支实现:
```
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int num = sc.nextInt();
if (num % 2 == 1) {
System.out.println(Math.sqrt(num));
} else {
System.out.println(Math.cbrt(num));
}
}
}
```
双分支实现:
```
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int num = sc.nextInt();
if (num % 2 == 1) {
System.out.println(Math.sqrt(num));
} else if (num % 2 == 0) {
System.out.println(Math.cbrt(num));
}
}
}
```
条件运算实现:
```
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int num = sc.nextInt();
String result = (num % 2 == 1) ? String.valueOf(Math.sqrt(num)) : String.valueOf(Math.cbrt(num));
System.out.println(result);
}
}
```
阅读全文
相关推荐
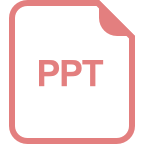
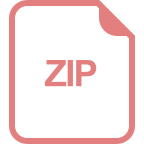
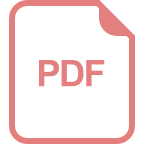













