服装销售管理系统 c++
时间: 2024-12-26 09:21:53 浏览: 3
### 使用 C++ 开发服装销售管理系统
#### 设计要求
构建一个完整的服装销售管理系统涉及多个方面的要求。系统应具备商品管理、库存控制、订单处理等功能,以满足日常运营需求[^1]。
#### 系统架构概述
为了确保系统的高效性和可维护性,在设计之初需考虑模块化结构。主要分为数据层、业务逻辑层以及表示层三个部分:
- **数据层**:负责存储和检索所有必要的商业信息;
- **业务逻辑层**:定义具体的操作流程和服务接口;
- **表示层**:提供给用户的交互界面;
#### 关键功能实现
##### 商品管理模块
此模块允许管理员录入新商品的信息并对其进行分类管理和查询操作。
```cpp
#include <iostream>
#include <vector>
using namespace std;
struct Product {
int id;
string name;
double price;
};
class InventoryManager {
public:
void addProduct(const Product& product);
bool removeProduct(int productId);
const vector<Product>& getProducts() const { return products_; }
private:
vector<Product> products_;
};
```
##### 库存监控机制
通过设置阈值来自动提醒补货情况,并记录每次进货的数量变化。
```cpp
void checkStockLevel(Product* p, int threshold) {
if (p->quantity <= threshold) {
cout << "Warning: Low stock level for item ID:" << p->id << endl;
}
}
```
##### 销售交易处理
支持创建新的销售单据,计算总价,并更新相应产品的剩余数量。
```cpp
double calculateTotal(vector<int> itemsIdList, map<int, Product*> productList){
double total = 0.0f;
for(auto itemId : itemsIdList){
auto it = productList.find(itemId);
if(it != productList.end()){
total += (*it).second->price;
}else{
cerr<<"Item not found!"<<endl;
}
}
return total;
}
```
#### 用户体验优化建议
考虑到实际应用场景中的便利性和友好度,可以加入图形化的前端展示方式,比如利用Qt框架快速搭建GUI应用[^3]。
阅读全文
相关推荐
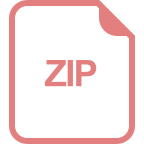
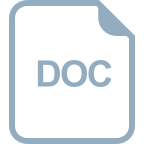
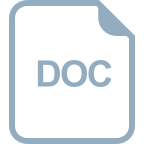
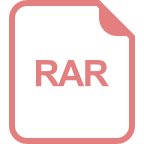
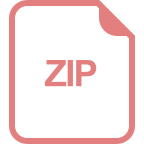
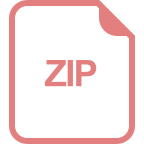
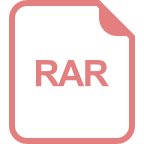
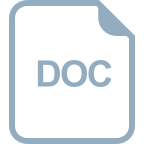
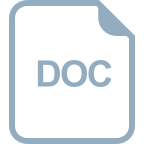
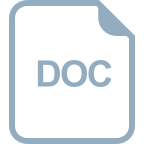
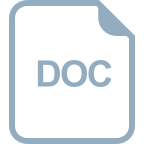
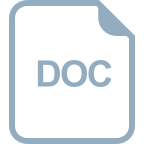
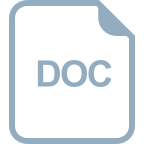
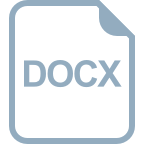
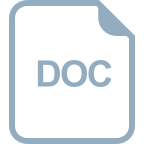
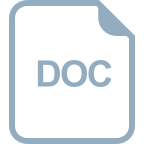