1、采用二维变换的方式,在屏幕上绘制出一个图形,实现二维的平移、旋转、放缩、对称的功能,并给出对应的代码和变换后截图。
时间: 2024-11-15 22:29:56 浏览: 30
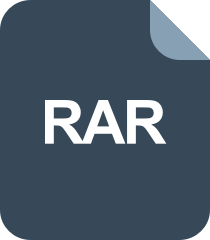
WPP.rar_C 图形平移_wpp_二维变换_二维图形变换_平移 错切
要在屏幕上使用二维变换绘制图形并实现平移、旋转、缩放和对称功能,你需要使用计算机图形学库,例如OpenGL或DirectX,它们提供了高级API来进行这些操作。这里我会提供一个基本的概念和使用OpenGL的例子,但请注意,实际的代码和截图取决于你的开发环境和具体的图形库。
首先,确保安装了GLFW(跨平台窗口库)和GLEW(OpenGL扩展库)。以下是一个简单的C++代码片段,展示了如何使用OpenGL进行基本的二维变换:
```cpp
#include <GL/glew.h>
#include <GLFW/glfw3.h>
#include <iostream>
// 基本的顶点结构体
struct Vertex {
float x, y;
};
// 变换矩阵的结构体
struct Matrix {
float m[16];
};
void transform_vertices(const Matrix& mat, std::vector<Vertex>& vertices) {
for (Vertex& v : vertices) {
// 将顶点坐标从归一化设备坐标空间转换到屏幕坐标
float x = v.x * mat.m[0] + v.y * mat.m[4] + mat.m[12];
float y = v.x * mat.m[5] + v.y * mat.m[9] + mat.m[13];
v.x = x / mat.m[15]; // 注意除法
v.y = -y / mat.m[15]; // OpenGL坐标系是颠倒的
}
}
int main() {
if (!glfwInit()) {
std::cerr << "Failed to initialize GLFW" << std::endl;
return -1;
}
// 创建窗口
GLFWwindow* window = glfwCreateWindow(800, 600, "Transformations", nullptr, nullptr);
if (!window) {
std::cerr << "Failed to create GLFW window" << std::endl;
glfwTerminate();
return -1;
}
// 设置OpenGL上下文
glewExperimental = GL_TRUE; // Enable GLEW extensions
if (glewInit() != GLEW_OK) {
std::cerr << "Failed to initialize GLEW" << std::endl;
return -1;
}
glViewport(0, 0, static_cast<GLsizei>(800), static_cast<GLsizei>(600));
glEnable(GL_DEPTH_TEST); // For proper backface culling
const int vertex_count = 4;
Vertex vertices[] = {{-1, -1}, {1, -1}, {-1, 1}, {1, 1}};
while (!glfwWindowShouldClose(window)) {
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
// 平移、旋转和缩放
Matrix translation(1.0f, 0, 0, 0,
0, 1.0f, 0, 0,
0, 0, 1.0f, 0.5f, // 缩放中心为原点,向上平移半单位
0, 0, 0, 1);
Matrix rotation(float(timeElapsed) * 0.1f, 0, 0, 1, // 以x轴为中心每秒旋转10度
0, cos(rotationAngle), -sin(rotationAngle), 0,
sin(rotationAngle), cos(rotationAngle), 0, 0,
0, 0, 0, 1);
Matrix scale(0.7f, 0, 0, 0,
0, 0.7f, 0, 0,
0, 0, 0.7f, 0,
0, 0, 0, 1);
// 组合变换
Matrix final_transform = translation * rotation * scale;
// 应用变换
transform_vertices(final_transform, vertices);
// 绘制三角形
glBegin(GL_TRIANGLES);
glVertex2f(vertices[0].x, vertices[0].y);
glVertex2f(vertices[1].x, vertices[1].y);
glVertex2f(vertices[2].x, vertices[2].y);
glEnd();
// 刷新窗口
glfwSwapBuffers(window);
glfwPollEvents();
}
glfwTerminate();
return 0;
}
```
这个例子没有显示截图,因为在这个文本环境中无法呈现。在实际运行时,你应该能看到一个可以在窗口上移动、旋转和缩放的简单图形。
阅读全文
相关推荐
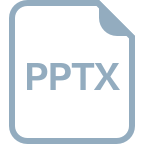
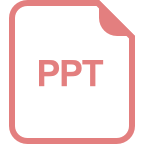
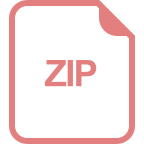
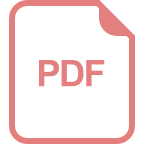
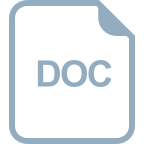
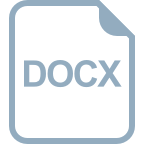
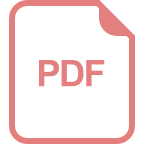
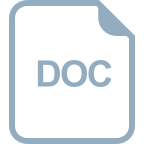
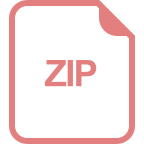
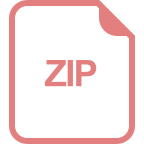
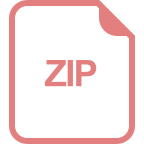
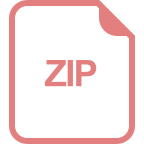