如何用Python的Tkinter库实现一个完整的石头剪刀布游戏界面并包含用户输入和电脑随机选择功能?
时间: 2024-12-11 13:44:20 浏览: 25
要使用Python的Tkinter库创建一个简单的石头、剪刀、布游戏界面,首先需要安装Tkinter库,如果还没有安装,可以使用`pip install tkinter`命令。以下是实现这个游戏的基本步骤:
1. 导入所需模块:
```python
import tkinter as tk
import random
```
2. 定义游戏选项和用户输入的变量:
```python
choices = ['石头', '剪刀', '布']
user_choice = None
computer_choice = None
```
3. 创建主窗口和布局:
```python
root = tk.Tk()
root.title("石头剪刀布")
root.geometry('400x300')
```
添加标签、按钮以及结果显示区域:
```python
tk.Label(root, text="请选择:").grid(row=0, column=1)
rock_button = tk.Button(root, text='石头', command=lambda: user_choice('石头'))
rock_button.grid(row=1, column=1)
scissors_button = tk.Button(root, text='剪刀', command=lambda: user_choice('剪刀'))
scissors_button.grid(row=2, column=1)
paper_button = tk.Button(root, text='布', command=lambda: user_choice('布'))
paper_button.grid(row=3, column=1)
result_label = tk.Label(root, text="")
result_label.grid(row=4, columnspan=2)
```
4. 定义用户选择函数,这里使用lambda表达式捕获按钮点击事件:
```python
def user_choice(choice):
global user_choice
user_choice = choice
if user_choice is not None:
play_game()
```
5. 定义计算机选择和游戏结果计算:
```python
def play_game():
computer_choice = random.choice(choices)
result = compare_choices(user_choice, computer_choice)
result_label.config(text=f"你选了{user_choice},电脑选了{computer_choice},结果是:{result}")
```
6. 比较用户和电脑的选择,并给出胜负判断:
```python
def compare_choices(player, opponent):
if player == opponent:
return "平局"
elif (player == '石头' and opponent == '剪刀') or (player == '剪刀' and opponent == '布') or (player == '布' and opponent == '石头'):
return "你赢了!"
else:
return "你输了!"
# 添加游戏开始和结束的逻辑(如按钮或计时器)
```
7. 运行主循环:
```python
root.mainloop()
```
阅读全文
相关推荐
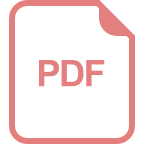
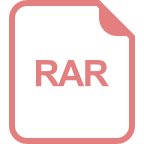
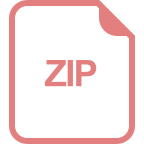















