SMC1602a液晶屏静态显示1到8的c语言全部代码
时间: 2024-09-26 20:13:15 浏览: 29
SMC1602A是一种常见的16×2字符液晶模块,它需要配合I2C总线通信来控制。以下是使用C语言通过I2C接口驱动SMC1602A液晶屏静态显示数字1到8的基本步骤。这里假设你已经包含了必要的头文件并初始化了I2C通信:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/ioctl.h>
#include <linux/i2c-dev.h>
#define LCD_I2C_ADDR 0x27 // SM1602A的地址通常为0x27或0x3F
// LCD指令集
#define LCD_CLEARDISPLAY 0x01
#define LCD_RETURN_HOME 0x02
#define LCD_ENTRY_MODE_SET 0x04
#define LCD_DISPLAY_ON 0x08
#define LCD_CURSOR_ON 0x0C
#define LCD_BLINK_ON 0x0F
#define LCD_LINE_1 0x80
#define LCD_LINE_2 0xC0
void writeCommand(uint8_t command) {
int fd = open("/dev/i2c-1", O_RDWR); // 打开I2C设备
if (fd == -1) {
perror("Failed to open I2C device");
return;
}
uint8_t data[2] = {command, 0}; // 数据包,包含命令和停止信号
i2c_write(fd, &data, sizeof(data)); // 发送数据
close(fd);
}
void writeData(uint8_t data) {
int fd = open("/dev/i2c-1", O_RDWR);
if (fd == -1) {
perror("Failed to open I2C device");
return;
}
uint8_t dataOut[] = {LCD_LINE_1, data};
i2c_write(fd, dataOut, sizeof(dataOut)); // 发送数据
close(fd);
}
void displayNumber(int number) {
switch (number) {
case 1: writeData('1'); break;
case 2: writeData('2'); break;
case 3: writeData('3'); break;
case 4: writeData('4'); break;
case 5: writeData('5'); break;
case 6: writeData('6'); break;
case 7: writeData('7'); break;
case 8: writeData('8'); break;
default: break; // 如果数字不在范围内,不做操作
}
}
int main() {
// 清除屏幕,回到起始位置,设置显示模式等
writeCommand(LCD_CLEARDISPLAY | LCD_ENTRY_MODE_SET | LCD_CURSOR_ON | LCD_DISPLAY_ON);
for (int i = 1; i <= 8; i++) {
displayNumber(i);
usleep(500000); // 等待一段时间再切换下一个数字,防止闪烁
}
return 0;
}
```
**注意:**
1. 这段代码假设你的系统上已经安装了I2C库,并且I2C总线设备名是/dev/i2c-1。
2. 根据你的硬件配置,I2C地址可能会有所不同,记得替换`LCD_I2C_ADDR`。
3. `i2c_write()`函数是一个简化的模拟,实际项目中可能需要根据你的I2C库实现替换。
相关推荐
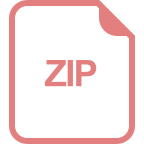
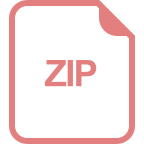
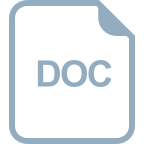














