python读取文件夹中图片名字
时间: 2023-11-03 10:07:06 浏览: 125
要读取文件夹中的图片文件名,可以使用Python中的os模块和os.listdir()方法。以下是一个示例代码:
```
import os
path = "path/to/your/folder"
image_extensions = ['.jpg', '.jpeg', '.png', '.gif']
for filename in os.listdir(path):
if os.path.splitext(filename)[1].lower() in image_extensions:
print(filename)
```
在这个示例代码中,首先定义了文件夹的路径和图片文件的扩展名列表。然后使用os.listdir()方法获取文件夹中的所有文件名,并使用for循环遍历这个列表。在循环中,使用os.path.splitext()方法来获取文件名和扩展名,并使用lower()方法将扩展名转换为小写字母。最后,使用if语句判断文件的扩展名是否在图片文件的扩展名列表中,如果是,则打印文件名。
相关问题
python读取文件夹中所有图片
可以使用Python的os、glob或者pathlib模块来实现。以下是使用os模块的示例代码:
```python
import os
folder_path = '/path/to/folder' # 文件夹路径
# 遍历文件夹中的所有文件和子文件夹
for root, dirs, files in os.walk(folder_path):
# 遍历当前文件夹中的所有文件
for file in files:
# 如果是图片文件
if file.endswith('.jpg') or file.endswith('.png'):
# 处理该图片文件,例如读取其内容等等
file_path = os.path.join(root, file) # 图片文件路径
# TODO: 处理该图片文件
```
该代码会遍历文件夹中所有的文件和子文件夹,如果该文件是图片文件(以".jpg"或".png"结尾),则处理该文件。你可以在TODO的位置写入处理代码。
python读取文件夹最新图片
要读取文件夹中的最新图片,你可以使用以下步骤:
1. 导入必要的模块:
```python
import os
```
2. 指定要读取的文件夹路径:
```python
folder_path = 'your_folder_path'
```
3. 获取文件夹中所有文件的列表,并根据最后修改时间进行排序:
```python
file_list = os.listdir(folder_path)
file_list.sort(key=lambda x: os.path.getmtime(os.path.join(folder_path, x)))
```
4. 确定最新图片的路径:
```python
latest_image = os.path.join(folder_path, file_list[-1])
```
现在,`latest_image` 就包含了文件夹中最新图片的完整路径。你可以根据需要进一步处理该文件。请确保将 "your_folder_path" 替换为实际的文件夹路径。
阅读全文
相关推荐
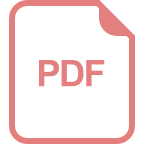













