写一个Java程序,实现以下要求: 学生参加某课程的考试,课程类型分为考试课和考查课 考试课最终成绩计算方法:出勤*10%+作业*10%+实验*10%+期末*70% 考查课最终成绩计算方法:出勤*20%+作业*10%+实验*10%+期末*60%
时间: 2024-09-26 20:00:51 浏览: 23
你可以创建一个名为`Course`的抽象类作为课程的基础类型,然后为`ExamCourse`和`CreditCourse`分别作为具体的子类来实现不同的成绩计算方式。以下是一个简单的示例:
```java
// 定义抽象类 Course
abstract class Course {
protected String name;
protected int attendance;
protected int homework;
protected int experiment;
protected int finalExam;
public Course(String name, int attendance, int homework, int experiment, int finalExam) {
this.name = name;
this.attendance = attendance;
this.homework = homework;
this.experiment = experiment;
this.finalExam = finalExam;
}
// 普通方法计算总成绩,子类需要覆盖这个方法
public abstract double calculateTotalScore();
// 公共方法供外部获取信息
public String getName() {
return name;
}
}
// 实现 ExamCourse 类型
class ExamCourse extends Course {
@Override
public double calculateTotalScore() {
return (attendance * 0.1) + (homework * 0.1) + (experiment * 0.1) + (finalExam * 0.7);
}
}
// 实现 CreditCourse 类型
class CreditCourse extends Course {
@Override
public double calculateTotalScore() {
return (attendance * 0.2) + (homework * 0.1) + (experiment * 0.1) + (finalExam * 0.6);
}
}
public class Main {
public static void main(String[] args) {
ExamCourse examCourse = new ExamCourse("Java", 90, 85, 92, 88);
System.out.println("Exam Course Name: " + examCourse.getName());
System.out.println("Total Score: " + examCourse.calculateTotalScore());
CreditCourse creditCourse = new CreditCourse("Maths", 95, 80, 87, 82);
System.out.println("\nCredit Course Name: " + creditCourse.getName());
System.out.println("Total Score: " + creditCourse.calculateTotalScore());
}
}
```
相关推荐
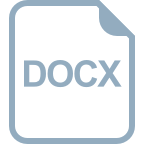
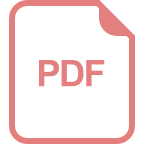
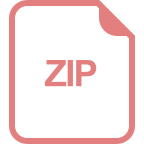














