写一个Java程序,实现以下要求: 学生参加某课程的考试,课程类型分为考试课和考查课 考试课最终成绩计算方法:出勤*10%+作业*10%+实验*10%+期末*70% 考查课最终成绩计算方法:出勤*20%+作业*10%+实验*10%+期末*60%
时间: 2024-09-25 17:01:18 浏览: 71
在Java中,我们可以创建一个`Course`抽象类和两个具体的子类`ExamCourse`和`AssessmentCourse`来满足这个需求。首先定义基础结构:
```java
public abstract class Course {
protected String courseName;
// 其他通用属性
public abstract double calculateFinalScore();
}
// 考试课子类
public class ExamCourse extends Course {
private int attendance;
private int homework;
private int experiment;
private int finalExam;
public ExamCourse(String courseName, int attendance, int homework, int experiment, int finalExam) {
this.courseName = courseName;
this.attendance = attendance;
this.homework = homework;
this.experiment = experiment;
this.finalExam = finalExam;
}
@Override
public double calculateFinalScore() {
return (attendance * 0.1) + (homework * 0.1) + (experiment * 0.1) + (finalExam * 0.7);
}
}
// 考查课子类
public class AssessmentCourse extends Course {
private int attendance;
private int homework;
private int experiment;
private int finalExam;
public AssessmentCourse(String courseName, int attendance, int homework, int experiment, int finalExam) {
this.courseName = courseName;
this.attendance = attendance;
this.homework = homework;
this.experiment = experiment;
this.finalExam = finalExam;
}
@Override
public double calculateFinalScore() {
return (attendance * 0.2) + (homework * 0.1) + (experiment * 0.1) + (finalExam * 0.6);
}
}
```
然后你可以通过创建实例并调用`calculateFinalScore`方法来计算学生的成绩:
```java
public static void main(String[] args) {
ExamCourse examCourse = new ExamCourse("数学", 90, 85, 92, 95);
System.out.println("考试课最终成绩: " + examCourse.calculateFinalScore());
AssessmentCourse assessmentCourse = new AssessmentCourse("英语", 95, 80, 88, 82);
System.out.println("考查课最终成绩: " + assessmentCourse.calculateFinalScore());
}
```
阅读全文
相关推荐
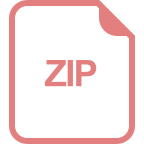
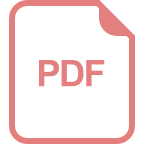
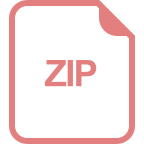


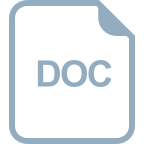
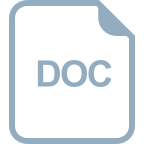
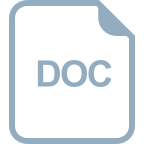
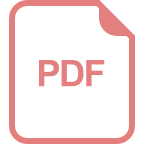
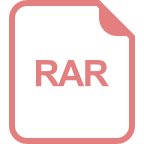
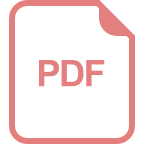
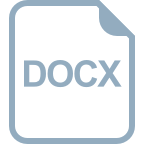
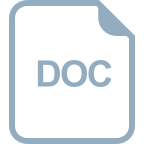
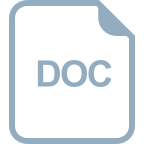
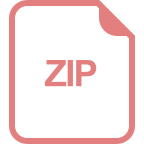