C语言线程入口函数怎么取参数
时间: 2024-10-09 07:15:42 浏览: 36
在C语言中,线程的入口函数通常是一个`void *`类型的指针,这个指针指向的是`pthread_start_routine`,也就是线程开始执行的函数。如果你想要在线程启动时传递参数,你需要将参数打包成一个结构体,并将其地址作为`void *`传入。
例如,假设你有一个结构体`ThreadParams`:
```c
typedef struct {
int value; // 你要传递的参数
} ThreadParams;
void *thread_entry(void *arg) {
ThreadParams *params = (ThreadParams *) arg;
printf("Received parameter: %d\n", params->value);
// 在这里处理参数...
}
```
然后,在创建线程的地方,你可以这样做:
```c
ThreadParams params = {42}; // 假设你要传递的值是42
pthread_create(&thread_id, ...,
thread_entry, ¶ms); // 参数就是params的地址
```
在这个例子中,`thread_entry`函数会通过`arg`接收并访问到`ThreadParams`中的数据。
相关问题
c语言线程与任务调度
在 C 语言中,线程是通过操作系统提供的线程库来实现的。线程可以让程序同时执行多个任务,从而提高程序的并发性和效率。
任务调度是指操作系统根据一定的策略将 CPU 的执行时间分配给不同的线程或进程。在 C 语言中,任务调度是由操作系统完成的,程序员只需要编写线程的代码,然后将线程交给操作系统进行调度。
通常情况下,线程的调度是基于优先级的。操作系统会将 CPU 时间分配给具有最高优先级的线程,直到该线程完成或被阻塞。当该线程完成或被阻塞后,操作系统会选择下一个具有最高优先级的线程运行。如果没有线程处于运行状态,那么操作系统会将 CPU 时间分配给等待队列中的线程。
线程在 C 语言中的使用需要包含头文件 pthread.h,并使用 pthread_create() 函数创建线程,该函数会返回一个线程 ID,然后使用 pthread_join() 函数等待线程结束。
下面是一个简单的例子,它创建了两个线程并让它们同时执行:
```
#include <stdio.h>
#include <pthread.h>
void* thread_function(void* arg)
{
printf("Thread %d is running\n", *((int*)arg));
return NULL;
}
int main()
{
pthread_t thread1, thread2;
int thread1_id = 1, thread2_id = 2;
pthread_create(&thread1, NULL, thread_function, &thread1_id);
pthread_create(&thread2, NULL, thread_function, &thread2_id);
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
return 0;
}
```
在上面的例子中,我们首先定义了一个函数 thread_function(),它将作为线程的入口点。然后我们使用 pthread_create() 函数创建了两个线程,并将它们分别赋值给 thread1 和 thread2 变量。每个线程都将调用 thread_function() 函数,并传递一个整数参数作为线程 ID。最后,我们使用 pthread_join() 函数等待线程结束。
C语言创建多线程运行一个函数并且函数中能控制关闭退出进程
在C语言中,可以使用线程库来创建多线程并运行一个函数。同时,可以通过在函数中使用全局变量或信号量等机制来控制线程的关闭和退出进程。
下面是一个示例代码,其中创建了一个新的线程,运行名为`myThreadFunc`的函数,并在函数中增加了一个全局变量`g_isRunning`来控制线程的退出:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
int g_isRunning = 1;
void* myThreadFunc(void* arg) {
while (g_isRunning) {
// 线程运行的代码
printf("This is a running thread.\n");
}
printf("Thread is exiting...\n");
pthread_exit(NULL);
}
int main() {
pthread_t myThread;
int ret = pthread_create(&myThread, NULL, myThreadFunc, NULL);
if (ret != 0) {
printf("Error: Failed to create thread.\n");
exit(EXIT_FAILURE);
}
// 主线程执行的代码
printf("Main thread is running...\n");
// 等待一段时间后关闭线程
sleep(5);
g_isRunning = 0;
// 等待线程退出
pthread_join(myThread, NULL);
printf("All threads are exited.\n");
// 退出进程
exit(EXIT_SUCCESS);
}
```
在上面的代码中,首先创建了一个新的线程,并将`myThreadFunc`函数作为线程的入口点。在`myThreadFunc`函数中,使用了一个循环来持续执行线程的代码,同时判断全局变量`g_isRunning`的值来控制线程的退出。在`main`函数中,输出了一条信息表示主线程正在运行,并在等待5秒后将`g_isRunning`设置为0来关闭线程。最后,使用`pthread_join`函数等待线程退出,并输出一条消息表示所有线程都已退出。最后,调用`exit`函数来结束进程。
阅读全文
相关推荐
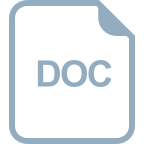
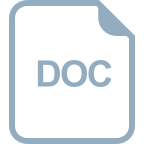
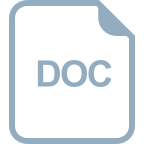
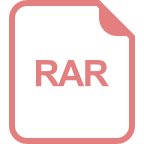
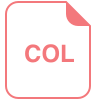
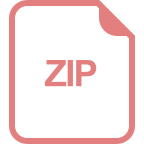
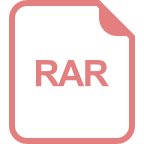
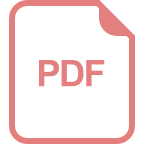
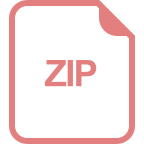
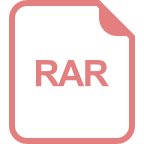
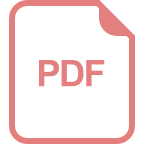
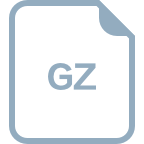
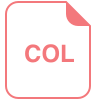
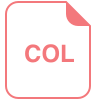
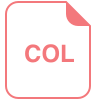

