windows下c语言多线程
时间: 2024-10-19 15:13:40 浏览: 36
在Windows下使用C语言进行多线程编程通常涉及以下几个步骤:
1. **包含头文件**:首先需要#include `<windows.h>`,因为其中包含了处理线程的相关函数。
2. **创建线程**:使用`CreateThread()`函数来创建一个新的线程。这个函数需要一个线程函数指针、线程堆栈大小和一些其他参数。
```c
HANDLE hThread = CreateThread(NULL, 0, MyThreadFunction, arg1, 0, NULL);
```
这里的`MyThreadFunction`是你为新线程指定的入口点函数,arg1是传递给该函数的参数。
3. **线程函数**:线程函数通常是`void*`类型的,你需要显式转换为具体的函数类型并调用实际的函数。例如:
```c
DWORD WINAPI MyThreadFunction(LPVOID lpParam) {
// 线程代码...
}
```
4. **同步与通信**:Windows提供了一些同步工具如互斥量(Mutex)、事件(Event)等,用于控制线程间的协作和数据访问。
5. **销毁线程**:当不需要线程时,可以使用`CloseHandle()`关闭线程句柄,但通常系统会在进程结束时自动回收未关闭的线程。
6. **错误处理**:记得检查`CreateThread()`的返回值,如果失败了可能需要捕获异常或处理错误。
相关问题
c语言多线程
C语言中的多线程可以通过使用线程库来实现,比如POSIX线程库(pthread)和Windows线程库。以下是一个简单的例子,展示如何使用pthread库创建和使用线程:
```c
#include <stdio.h>
#include <pthread.h>
void *my_thread(void *arg) {
int thread_index = *(int *)arg;
printf("This is thread #%d\n", thread_index);
pthread_exit(NULL);
}
int main() {
pthread_t threads[5];
int thread_args[5];
// 创建5个线程
for (int i = 0; i < 5; i++) {
thread_args[i] = i;
pthread_create(&threads[i], NULL, my_thread, (void *)&thread_args[i]);
}
// 等待线程结束
for (int i = 0; i < 5; i++) {
pthread_join(threads[i], NULL);
}
printf("All threads have finished execution\n");
return 0;
}
```
在上述代码中,我们使用了pthread库创建了5个线程,并在每个线程中输出了线程编号。在主函数中,我们使用pthread_join函数等待所有线程结束后再输出一条信息。
C语言多线程程序
在C语言中,可以使用多线程来实现在一个程序中同时执行多个任务的目的。C语言中实现多线程需要使用线程库,常见的线程库有POSIX线程库和Windows线程库。下面以POSIX线程库为例,介绍如何在C语言中编写多线程程序。
首先需要包含头文件pthread.h,然后使用pthread_create函数创建一个新线程,函数的语法如下:
```
int pthread_create(pthread_t *thread, const pthread_attr_t *attr,
void *(*start_routine)(void *), void *arg);
```
其中,thread是指向线程标识符的指针,attr是指向线程属性的指针,start_routine是指向线程函数的指针,arg是传递给线程函数的参数。
线程函数的形式如下:
```
void *thread_func(void *arg);
```
其中,arg是传递给线程函数的参数。
下面是一个简单的多线程程序示例:
```
#include <stdio.h>
#include <pthread.h>
void *thread_func(void *arg)
{
int i;
for (i = 0; i < 10; i++) {
printf("Thread %d: %d\n", *((int *)arg), i);
}
return NULL;
}
int main()
{
pthread_t thread1, thread2;
int arg1 = 1, arg2 = 2;
pthread_create(&thread1, NULL, thread_func, &arg1);
pthread_create(&thread2, NULL, thread_func, &arg2);
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
return 0;
}
```
上述代码创建了两个线程,分别执行thread_func函数,并传递不同的参数(1和2)给每个线程。在thread_func函数中,使用for循环输出线程号和计数器的值。在main函数中,调用pthread_join函数等待线程执行完毕,并回收线程资源。
需要注意的是,在多线程程序中,多个线程共享进程的内存空间,因此需要注意线程之间的同步和互斥问题,以避免线程间的竞争和冲突。
阅读全文
相关推荐
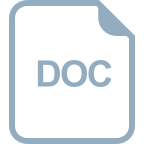
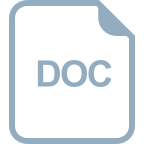
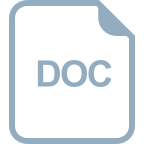
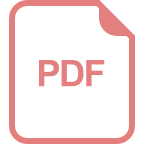
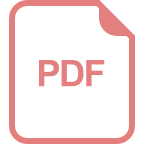
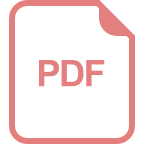
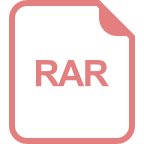
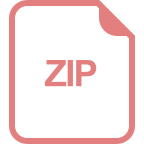
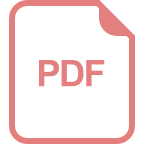
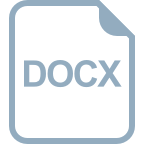
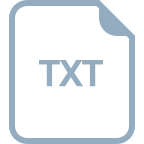
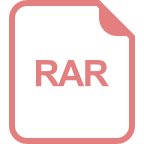
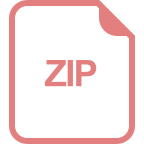
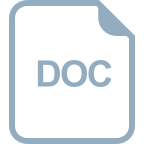
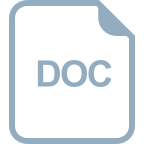
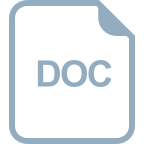
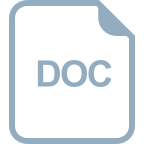