使用 Thread类创建子线程,并在子线程中显示“threadisrunning!”,使用sleep()方法 挂起5s,使用notify()方法唤醒进程,然后使用stop()方法撤销。
时间: 2024-09-26 16:14:03 浏览: 98
在Java中,你可以通过继承Thread类或者实现Runnable接口来创建子线程。下面是一个简单的示例,展示如何创建一个子线程并在其中实现你需要的功能:
```java
public class MyRunnable implements Runnable {
private boolean isRunning = true;
public void run() {
while (isRunning) {
System.out.println("threadisrunning!");
try {
// 挂起5秒
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
// 添加一个通知方法,用于唤醒线程
public synchronized void stopRunning() {
isRunning = false;
notify(); // 唤醒正在等待的线程
}
// 添加一个方法,用于启动线程
public void start() {
new Thread(this).start(); // 创建并启动线程
}
// 为了演示如何使用stop(),虽然这通常不是一个好的实践,因为它会立即终止线程,可能会导致未完成的操作
public void stop() {
if (!Thread.currentThread().equals(this)) { // 防止外部线程调用停止当前运行的线程
this.stopRunning();
try {
// 这里需要等待子线程结束
join(); // 如果你想让主线程等到子线程真正停止
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
// 主程序中使用
public static void main(String[] args) {
MyRunnable myRunnable = new MyRunnable();
myRunnable.start();
// 模拟延迟5秒后停止子线程
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
myRunnable.stop(); // 调用stop方法
}
```
在这个例子中,`run()`方法会在子线程中不断打印"threadisrunning!",直到`stopRunning()`方法被调用,将`isRunning`设置为`false`并唤醒等待的线程。然后,如果主线程尝试调用`stop()`,它会先调用`stopRunning()`,然后等待子线程结束。
阅读全文
相关推荐




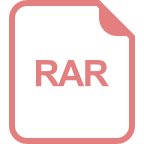

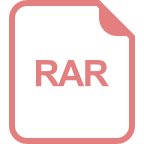



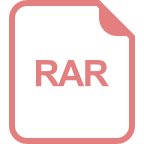

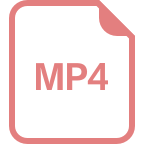
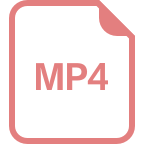



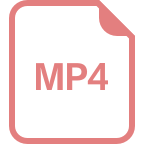