用Java数据结构的知识写一个算法,设线性表存于数组 a[0… n-1]的前R个分量中,且递增有序,试写一算法,将x插入 性表的适当位置上,以保持线性表的有序性。
时间: 2024-09-12 17:04:10 浏览: 45
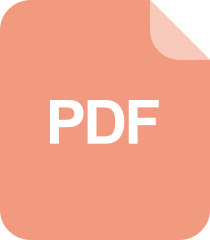
数据结构与算法java中文
在Java中,我们可以使用二分查找法以及插入操作来实现在有序数组`a[0...n-1]`中插入元素`x`并保持有序性的算法。这里是一个简单的步骤描述:
1. **初始化**:
- 定义两个指针`low`和`high`,初始值分别为0(表示左边界)和`n-1`(表示右边界)。
2. **二分查找**:
- 当`low <= high`时,执行循环:
- 计算中间索引`mid`:`mid = (low + high) / 2`。
- 比较`a[mid]`与`x`:
- 如果`a[mid] >= x`,说明我们需要在前半部分查找插入位置,更新`high = mid - 1`。
- 否则(即`a[mid] < x`),更新`low = mid + 1`。
3. **插入元素**:
- 当找到合适的插入位置,即`low == high`或`a[low] < x`时,将`x`插入到`a[low]`的位置:
- 将数组中的最后一个元素移到`low`位置:`a[low] = a[n-1]`。
- 变化数组长度`n -= 1`。
- 然后将`x`赋值给`a[low]`:`a[low] = x`。
4. **返回结果**:
- 插入完成后,算法结束,返回数组`a`。
以下是这个算法的伪代码形式:
```java
int binaryInsert(int[] a, int x, int R) {
int low = 0;
int high = R;
while (low <= high) {
int mid = (low + high) / 2;
if (a[mid] >= x) {
high = mid - 1;
} else {
low = mid + 1;
}
}
// Move the last element to make space for x
a[low] = a[R];
a[R] = x;
return low; // Return the index where x was inserted
}
```
阅读全文
相关推荐
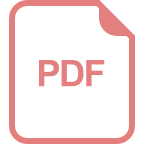
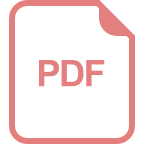
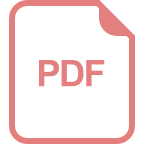
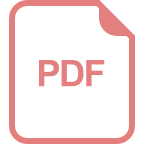
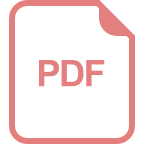
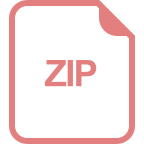
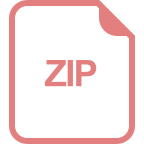
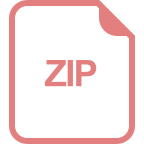
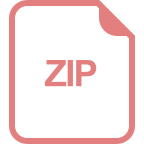
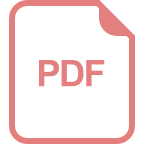
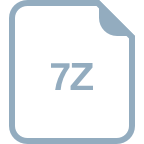
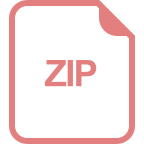
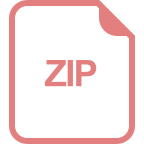
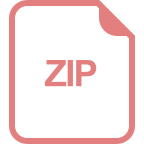
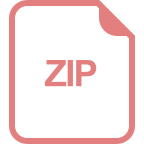
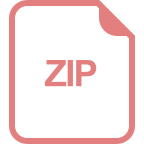