C++编写一个程序,设计学生信息的类,包含数据成员学号、姓名和成绩(语文、数学和英语)和总成绩,其中总成绩是各成绩之和。写出完整的类定义,并通过主函数中定义不同对象并实现该对象的信息输入、计算与输出。
时间: 2024-11-03 13:20:38 浏览: 11
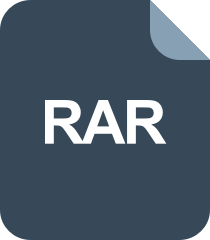
设计一个学生类Student(学生学号、姓名、数学、英语、计算机成绩;)

在C++中,你可以创建一个名为`Student`的类来表示学生信息。这个类会包含私有变量(数据成员)和公共方法(成员函数)来操作这些数据。下面是一个简单的例子:
```cpp
#include <iostream>
using namespace std;
class Student {
private:
int studentID;
string name;
int chineseScore;
int mathScore;
int englishScore;
public:
// 构造函数用于初始化学生的各项成绩
Student(int id, string n, int cs, int ms, int es) {
studentID = id;
name = n;
chineseScore = cs;
mathScore = ms;
englishScore = es;
}
// 计算总成绩
int calculateTotalScore() {
return chineseScore + mathScore + englishScore;
}
// 显示学生信息
void displayStudentInfo() {
cout << "学生ID: " << studentID << endl;
cout << "姓名: " << name << endl;
cout << "语文成绩: " << chineseScore << endl;
cout << "数学成绩: " << mathScore << endl;
cout << "英语成绩: " << englishScore << endl;
cout << "总成绩: " << calculateTotalScore() << endl;
}
};
int main() {
// 创建并初始化学生对象
Student s1(1001, "张三", 85, 92, 90);
Student s2(1002, "李四", 90, 88, 87);
// 输出学生s1的信息
s1.displayStudentInfo();
// 输出学生s2的信息
s2.displayStudentInfo();
return 0;
}
```
在这个例子中,我们首先定义了一个`Student`类,包含了学生的个人信息以及计算总成绩和显示信息的方法。然后,在`main`函数中,我们创建了两个`Student`对象`s1`和`s2`,分别设置了它们的数据,最后调用了各自的`displayStudentInfo`方法来展示信息。
阅读全文
相关推荐
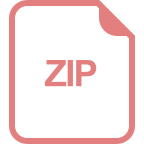
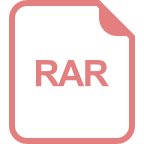







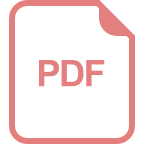
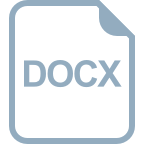
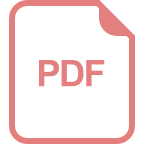
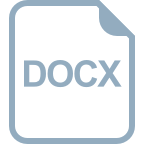

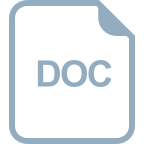
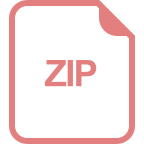
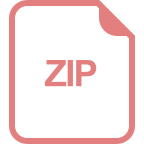
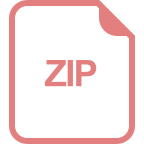
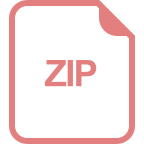