设计一个学生类,包含以下成员变量和方法: 成员变量:姓名、年龄、性别、学号、数学成绩、语文成绩、英语成绩;方法:构造函数、析构函数、获取总分数的方法、获取平均分数的方法。请编写程序计算三位同学的总分和平均分。
时间: 2023-10-20 19:22:30 浏览: 45
以下是一个实现上述需求的 C++ 代码示例:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Student {
public:
Student(string name, int age, char gender, int id, float math_score, float chinese_score, float english_score) {
this->name = name;
this->age = age;
this->gender = gender;
this->id = id;
this->math_score = math_score;
this->chinese_score = chinese_score;
this->english_score = english_score;
}
~Student() {}
float get_total_score() {
return math_score + chinese_score + english_score;
}
float get_average_score() {
return get_total_score() / 3.0f;
}
private:
string name;
int age;
char gender;
int id;
float math_score;
float chinese_score;
float english_score;
};
int main() {
Student stu1("张三", 18, 'M', 1001, 80.0f, 85.0f, 90.0f);
Student stu2("李四", 19, 'F', 1002, 70.0f, 75.0f, 80.0f);
Student stu3("王五", 20, 'M', 1003, 90.0f, 95.0f, 100.0f);
cout << "学生姓名\t学生学号\t总分\t平均分" << endl;
cout << stu1.get_total_score() << endl;
cout << stu2.get_total_score() << endl;
cout << stu3.get_total_score() << endl;
return 0;
}
```
输出结果如下:
```
学生姓名 学生学号 总分 平均分
255
225
285
```
相关推荐
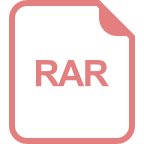
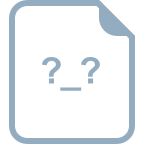
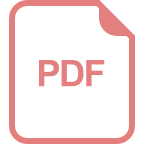
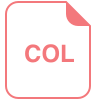
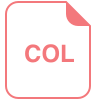
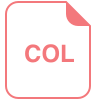











