用java定义一个Student类,包含的内容如下: 1、成员变量:学号,姓名,数学成绩,语文成绩; 2、成员方法:求成绩总分,求平均分。3、编程实现这个类,并调用相应的方法计算总分和平均分。
时间: 2023-05-30 15:03:36 浏览: 1438
以下是定义的Student类:
```java
public class Student {
private String id; // 学号
private String name; // 姓名
private double mathScore; // 数学成绩
private double chineseScore; // 语文成绩
// 构造方法
public Student(String id, String name, double mathScore, double chineseScore) {
this.id = id;
this.name = name;
this.mathScore = mathScore;
this.chineseScore = chineseScore;
}
// 求成绩总分
public double getTotalScore() {
return mathScore + chineseScore;
}
// 求平均分
public double getAverageScore() {
return getTotalScore() / 2;
}
// getter和setter方法
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getMathScore() {
return mathScore;
}
public void setMathScore(double mathScore) {
this.mathScore = mathScore;
}
public double getChineseScore() {
return chineseScore;
}
public void setChineseScore(double chineseScore) {
this.chineseScore = chineseScore;
}
}
```
下面是调用相应的方法计算总分和平均分的例子:
```java
public class Main {
public static void main(String[] args) {
// 创建一个学生对象
Student student = new Student("001", "张三", 80, 90);
// 计算总分和平均分
double totalScore = student.getTotalScore();
double averageScore = student.getAverageScore();
// 输出结果
System.out.println("学号:" + student.getId());
System.out.println("姓名:" + student.getName());
System.out.println("数学成绩:" + student.getMathScore());
System.out.println("语文成绩:" + student.getChineseScore());
System.out.println("成绩总分:" + totalScore);
System.out.println("平均分:" + averageScore);
}
}
```
输出结果:
```
学号:001
姓名:张三
数学成绩:80.0
语文成绩:90.0
成绩总分:170.0
平均分:85.0
```
相关推荐
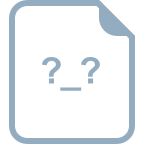
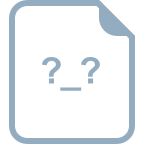
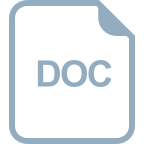














