用python设计一套程序,专门用在ZPW-2000A轨道电路图中,鼠标放在哪里就能显示出哪里的电压电流,给出代码
时间: 2024-09-10 20:28:38 浏览: 85
设计一个Python程序来实现在ZPW-2000A轨道电路图中的交互式电压和电流显示功能,可以结合PyQt库创建图形用户界面(GUI),并利用matplotlib库来绘制地图以及实时更新数据。这里提供一个基本的框架,具体的细节需要根据实际的电路图数据结构和数据库来完善:
```python
import sys
import matplotlib.pyplot as plt
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QWidget, QVBoxLayout, QGraphicsScene, QGraphicsView, QMouseEvent
from PyQt5.QtGui import QPainter, QColor
class CircuitMap(QGraphicsView):
def __init__(self, data, parent=None):
super().__init__(parent)
self.data = data
self.scene = QGraphicsScene()
self.setScene(self.scene)
self.update_map()
def update_map(self):
for location, (voltage, current) in self.data.items():
point = QGraphicsRectItem(*location, 20, 20) # 假设每个位置是一个20x20像素的矩形
point.setToolTip(f"Voltage: {voltage}, Current: {current}")
point.setBrush(QColor('blue')) # 可视化颜色仅作示例
point.hoverMoveEvent = self.on_hover_move
self.scene.addItem(point)
def on_hover_move(self, event):
pos = event.pos() # 获取鼠标在窗口的位置
# 在这个位置查找对应的数据,例如通过索引、坐标转换等
voltage, current = self.data.get_location_at(pos)
if voltage and current:
label = QLabel(f"Voltage: {voltage} V, Current: {current} A")
self.label = label
self.label.show()
self.label.move(event.globalPos()) # 将标签移动到鼠标位置
else:
if self.label:
self.label.hide()
if __name__ == "__main__":
app = QApplication(sys.argv)
circuit_data = {...} # 这里假设circuit_data是你从数据库或者其他源获取的实际电压电流数据
window = QMainWindow()
map_view = CircuitMap(circuit_data)
window.setCentralWidget(map_view)
window.show()
sys.exit(app.exec_())
```
阅读全文
相关推荐
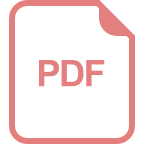
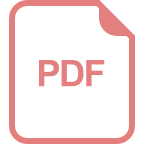
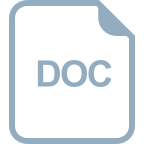
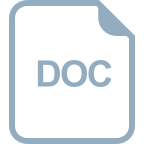
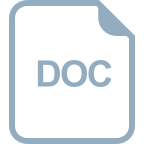
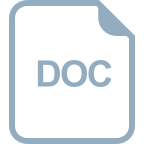

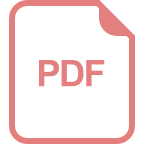
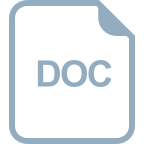





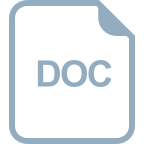
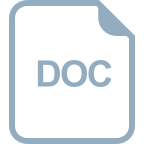
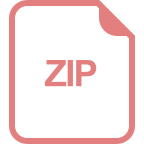