请在上述代码中不要使用#include <vector> #include <thread>
时间: 2024-12-24 14:23:05 浏览: 2
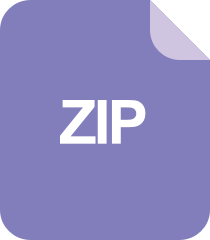
C++ 控制台 调用Timer,实现多线程
好的,如果你不想使用`<vector>`和`<thread>`头文件,我们可以直接用数组和`sleep()`函数来代替。这里是一个简化版的PCB数据结构和时间片轮转调度的代码:
```cpp
#include <iostream>
#include <cstdlib> // 使用这个库中的stdlib.h替换系统调用sleep()
// 简化版PCB数据结构
struct PCB {
int pid;
char* name;
bool running;
int priority;
int cpu_time;
// 功能函数的简单表示
void (*thread_func)();
};
// 使用数组模拟进程就绪队列
PCB processes[] = {
{1, "Process1", true, 5, 0, [](void){ std::cout << "Process1 running\n"; sleep(2); }},
{2, "Process2", false, 3, 0, [](void){ std::cout << "Process2 waiting\n"; }}
};
const size_t NUM_PROCESSES = sizeof(processes) / sizeof(processes[0]);
// 时间片轮转调度函数
void time_slice_round_robin(int quantum) {
for (size_t i = 0; i < NUM_PROCESSES; ++i) {
PCB& current = processes[i];
if (current.running) {
current.cpu_time += quantum;
if (current.cpu_time >= quantum) {
current.cpu_time = 0;
current.running = false;
// 比较优先级,这里简化为随机决定是否移动到队首
srand(time(0));
bool move_to_front = rand() % 2 == 1;
if (move_to_front && current.priority > processes[0].priority) {
swap(processes[i], processes[0]);
}
} else {
(*current.thread_func)();
}
}
}
}
int main() {
int quantum = 1; // 假设时间片长度为1秒
time_slice_round_robin(quantum);
return 0;
}
```
这段代码将进程和就绪队列改为了静态数组,并移除了`<vector>`和`<thread>`相关的引用。请注意,这只是一个非常基础的示例,实际上数组大小固定,不适合动态增加或删除进程,而且没有真正的并发支持,无法模拟真实的操作系统环境。
阅读全文
相关推荐
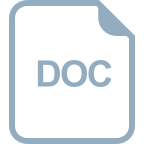
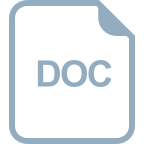







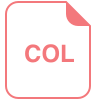
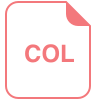
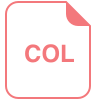





