写出判断三角形类型的c程序
时间: 2024-11-09 12:26:45 浏览: 7
确定一个三角形的类型(如等边、等腰或普通三角形)通常需要知道三边的长度。你可以根据以下条件编写一个C程序:
1. **等边三角形**:所有三条边都相等。
2. **等腰三角形**:至少有两条边相等。
3. **普通三角形**:三条边都不相等。
这里是一个简单的C程序示例,它使用`scanf`从用户那里获取三个边长,然后检查它们是否满足上述条件:
```c
#include <stdio.h>
// 判断三角形类型
enum TriangleType {
NOT_A_TRIANGLE,
Equilateral, // 等边三角形
Isosceles, // 等腰三角形
Scalene // 普通三角形
};
int main() {
float a, b, c;
printf("Enter the lengths of triangle sides (separated by space): ");
scanf("%f %f %f", &a, &b, &c);
if (a <= 0 || b <= 0 || c <= 0) { // 非正数边缘无法构成三角形
printf("Invalid input. Sides must be positive.\n");
return NOT_A_TRIANGLE;
}
// 检查边长关系
if (a == b && b == c) { // 等边三角形
printf("The triangle is equilateral.\n");
return Equilateral;
} else if (a == b || b == c || a == c) { // 等腰三角形
printf("The triangle is isosceles.\n");
return Isosceles;
} else { // 不等边三角形
printf("The triangle is scalene.\n");
return Scalene;
}
}
```
当你运行这个程序时,它会提示用户输入三个边长,然后输出三角形的类型。如果输入的边长不满足构成三角形的条件,它会显示错误信息。
阅读全文
相关推荐
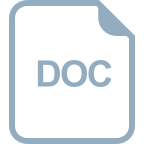
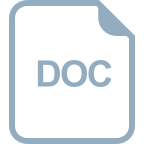
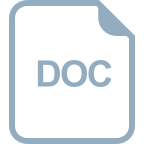


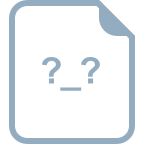












