如何用Java实现A*搜索算法的代码?
时间: 2024-10-23 21:03:43 浏览: 5
A*搜索算法是一种启发式搜索算法,常用于路径规划问题,特别是在游戏开发、机器人导航等领域。在Java中实现A*算法,通常需要以下步骤:
1. 定义节点类(Node),包含状态、代价(g值)、预计代价(h值)以及指向父节点的引用。
```java
public class Node {
private Position position;
private int gCost; // 实际成本
private int hCost; // 启发函数估计的成本
private Node parent;
// 构造函数等...
}
```
2. 创建开放列表(openList)和关闭列表(closedList)来存储待处理和已处理的节点。
3. 函数`aStarSearch(start, end)`,其中`start`是起点,`end`是目标点。
```java
List<Node> aStarSearch(Node start, Node goal) {
openList = new PriorityQueue<>((n1, n2) -> n1.getFValue() - n2.getFValue());
openList.add(start);
while (!openList.isEmpty()) {
Node current = openList.poll();
if (current.equals(goal)) {
return reconstructPath(current);
}
closedList.add(current);
for (Edge edge : current.getConnections()) {
Node neighbor = edge.getDestination();
if (closedList.contains(neighbor)) continue; // 已经访问过
neighbor.gCost = current.gCost + edge.getCost();
neighbor.hCost = heuristicFunction(neighbor.position, goal.position);
neighbor.parent = current;
// 检查邻居是否应该在openList中移动到前面
if (!openList.contains(neighbor) || neighbor.getFValue() < neighbor.getGValue() + neighbor.HValue) {
openList.removeIf(n -> n == neighbor);
openList.add(neighbor);
}
}
}
return null; // 如果找不到路径,返回null
}
// 哈希函数计算f值(总成本)
private int getFValue(Node node) {
return node.gCost + node.hCost;
}
// 重构路径
private List<Node> reconstructPath(Node current) {
List<Node> path = new ArrayList<>();
while (current != null) {
path.add(current);
current = current.parent;
}
Collections.reverse(path);
return path;
}
```
阅读全文
相关推荐
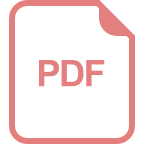
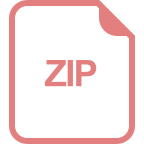
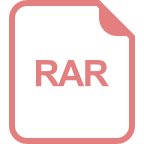
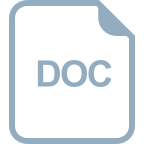
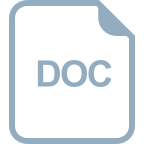

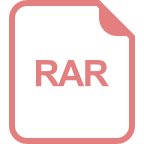
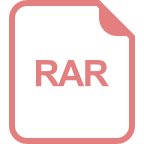
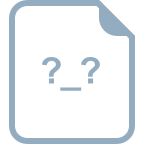
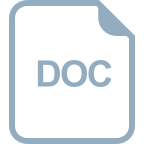
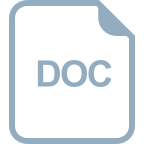
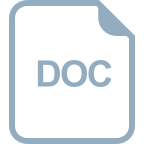
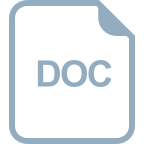
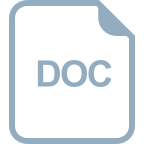
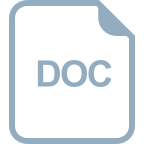
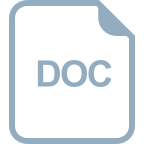
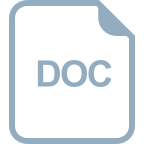
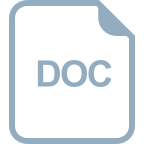
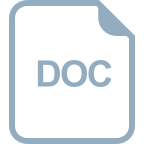