self.device = next(self.model.parameters()).device
时间: 2024-11-13 16:26:30 浏览: 8
这句话是在PyTorch编程中常见的操作,它表示将`self.model`模型中的第一个参数(假设这是一个张量)的数据所在的设备赋值给`self.device`变量。`next()`函数用于获取迭代器的下一个元素,这里通过`self.model.parameters()`得到模型的所有参数生成器,然后取第一个参数。这通常发生在需要将计算移动到GPU(如果有可用),以便利用其并行计算能力的时候。
例如,如果你有一个已经在GPU上训练的模型,你可能会这样做来保证所有的后续计算都在GPU上进行:
```python
if torch.cuda.is_available():
self.device = torch.device("cuda")
else:
self.device = torch.device("cpu")
self.model.to(self.device) # 将模型移到指定设备
self.device = next(self.model.parameters()).device # 获取设备
# 现在,所有对self.model的操作都将自动在self.device上执行
```
相关问题
class ComputeLoss: sort_obj_iou = False # Compute losses def __init__(self, model, autobalance=False): device = next(model.parameters()).device # get model device h = model.hyp # hyperparameters # Define criteria BCEcls = nn.BCEWithLogitsLoss(pos_weight=torch.tensor([h['cls_pw']], device=device)) BCEobj = nn.BCEWithLogitsLoss(pos_weight=torch.tensor([h['obj_pw']], device=device)) # Class label smoothing https://arxiv.org/pdf/1902.04103.pdf eqn 3 self.cp, self.cn = smooth_BCE(eps=h.get('label_smoothing', 0.0)) # positive, negative BCE targets # Focal loss g = h['fl_gamma'] # focal loss gamma if g > 0: BCEcls, BCEobj = FocalLoss(BCEcls, g), FocalLoss(BCEobj, g) m = de_parallel(model).model[-1] # Detect() module self.balance = {3: [4.0, 1.0, 0.4]}.get(m.nl, [4.0, 1.0, 0.25, 0.06, 0.02]) # P3-P7 self.ssi = list(m.stride).index(16) if autobalance else 0 # stride 16 index self.BCEcls, self.BCEobj, self.gr, self.hyp, self.autobalance = BCEcls, BCEobj, 1.0, h, autobalance self.na = m.na # number of anchors self.nc = m.nc # number of classes self.nl = m.nl # number of layers self.anchors = m.anchors self.device = device这个代码什么意思
这段代码是定义了一个 ComputeLoss 类,用于计算 YOLOv5 模型的损失值。在初始化时,会根据模型的超参数定义不同的损失函数,包括分类损失 BCEcls、目标检测损失 BCEobj 和 Focal Loss。同时还对正负样本进行了平滑处理,以及为不同的特征层定义了不同的权重平衡。在计算损失时,会用到模型的输出以及一些超参数,最终返回损失值。
用Python写一个使用冒号后面的类的MAML算法:class LSTMModel(nn.Module): def init(self, input_size, hidden_size, output_size, num_layers, look_back): super(LSTMModel, self).init() self.hidden_size = hidden_size self.num_layers = num_layers self.look_back = look_back self.lstm1 = nn.LSTM(input_size, hidden_size, num_layers, batch_first=True) self.lstm2 = nn.LSTM(hidden_size, hidden_size, num_layers, batch_first=True) self.fc = nn.Linear(hidden_size, output_size) def forward(self, x): h0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(device) c0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(device) x = x.view(-1, self.look_back, 1) out, _ = self.lstm1(x, (h0, c0)) out, _ = self.lstm2(out, (h0, c0)) out = self.fc(out[:, -1, :]) return out
MAML (Model-Agnostic Meta-Learning) 是一种元学习算法,可以在少量数据的情况下快速适应新任务。下面是使用 Python 实现的 MAML 算法:
```python
import torch
import torch.nn as nn
import torch.optim as optim
class MAML:
def __init__(self, model, loss_fn, lr_inner=0.1, lr_outer=0.001):
self.model = model
self.loss_fn = loss_fn
self.lr_inner = lr_inner
self.lr_outer = lr_outer
self.optimizer = optim.Adam(self.model.parameters(), lr=self.lr_outer)
def train(self, tasks, k=1, num_updates=1):
for task in tasks:
# Clone the model to avoid modifying the original model
model_copy = type(self.model)(**self.model_params)
model_copy.load_state_dict(self.model.state_dict())
# Create a new optimizer for the task
optimizer = optim.SGD(model_copy.parameters(), lr=self.lr_inner)
# Train the model on the task for `num_updates` steps
for i in range(num_updates):
# Compute the loss on the task
loss = self.loss_fn(model_copy(task['train']['x']), task['train']['y'])
# Compute the gradients
loss.backward()
# Update the model parameters with the inner optimizer
optimizer.step()
# Zero the gradients for the next iteration
optimizer.zero_grad()
# Compute the loss on the validation set
val_loss = self.loss_fn(model_copy(task['val']['x']), task['val']['y'])
# Compute the gradients of the validation loss w.r.t. the model parameters
val_loss.backward()
# Update the model parameters using the outer optimizer
self.optimizer.step()
# Zero the gradients for the next task
self.optimizer.zero_grad()
```
其中,`model` 是用于元学习的模型,`loss_fn` 是损失函数,`lr_inner` 和 `lr_outer` 分别是内部优化器和外部优化器的学习率。`train` 方法接受一个任务列表 `tasks`,每个任务包含训练集和验证集,并且在每个任务上执行 `k` 步内部更新和 `num_updates` 步外部更新。在每个任务上,我们首先复制模型并使用内部优化器训练模型,然后在验证集上计算损失并使用外部优化器更新模型参数。最后,我们将外部优化器的梯度归零,以准备处理下一个任务。
你提供的代码是一个 LSTM 模型,可以使用上述 MAML 类对其进行元学习。只需将 LSTM 模型传递给 `MAML` 类的构造函数即可。在 `train` 方法中,你需要将任务列表转换成适当的格式,并调用 `MAML` 类的 `train` 方法。下面是一个示例:
```python
# Create a MAML object
maml = MAML(LSTMModel(input_size, hidden_size, output_size, num_layers, look_back), nn.MSELoss())
# Define the tasks
tasks = [
{
'train': {'x': train_x1, 'y': train_y1},
'val': {'x': val_x1, 'y': val_y1}
},
{
'train': {'x': train_x2, 'y': train_y2},
'val': {'x': val_x2, 'y': val_y2}
},
...
]
# Train the model on the tasks
maml.train(tasks, k=1, num_updates=1)
```
在上面的代码中,我们使用 `LSTMModel` 类创建了一个 `MAML` 对象,并为每个任务定义了训练集和验证集。我们使用 `train` 方法训练模型,并指定 `k=1` 和 `num_updates=1`,这意味着我们在每个任务上执行一次内部更新和一次外部更新。你需要根据你的具体情况调整这些参数。
阅读全文
相关推荐
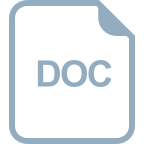
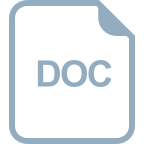
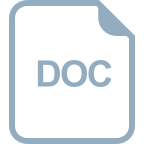

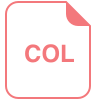
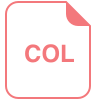


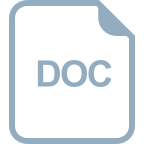
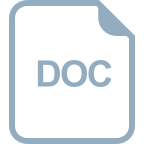
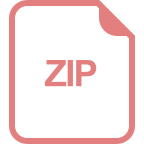
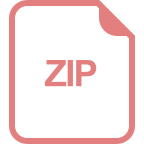
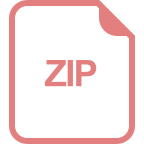