用winform实现跑马灯
时间: 2024-06-20 10:03:16 浏览: 176
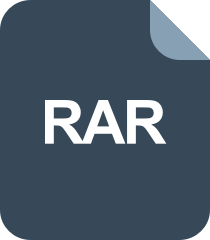
winform label 当label字体长度大于label宽度的时候 实现跑马灯效果 左右移动
WinForm是Windows Forms的缩写,它是一种用于创建桌面应用程序的.NET框架。在WinForm中,实现跑马灯可以通过使用Timer控件和Label控件来实现。
步骤如下:
1. 在WinForm中添加一个Label控件。
2. 设置Label控件的属性,例如Font、ForeColor、BackColor、Text等。
3. 在WinForm中添加一个Timer控件。
4. 设置Timer控件的Interval属性,表示跑马灯滚动的速度。
5. 在Timer的Tick事件中编写代码,实现Label控件的Text属性进行滚动。
以下是一个简单的示例代码:
```csharp
private void Form1_Load(object sender, EventArgs e)
{
label1.Text = "这是一条跑马灯公告!";
timer1.Interval = 100;
timer1.Enabled = true;
}
private void timer1_Tick(object sender, EventArgs e)
{
string temp = label1.Text.Substring(1) + label1.Text.Substring(0, 1);
label1.Text = temp;
}
```
阅读全文
相关推荐
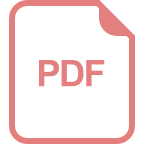





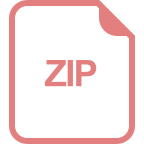
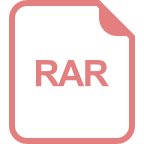
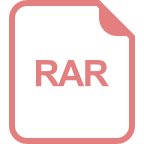
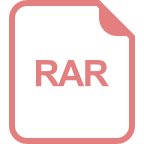
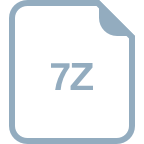
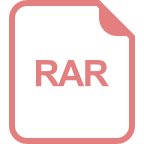
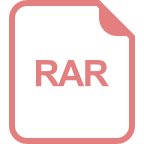
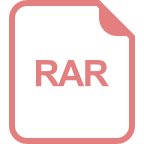
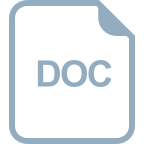
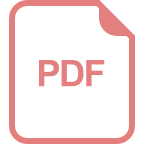