winform 做个跑马灯
时间: 2024-06-03 20:06:34 浏览: 13
Winform 是一种基于 Windows 操作系统的 GUI 应用程序框架,它提供了开发桌面应用程序所需的基本组件和控件。做一个跑马灯,可以使用 Winform 中的 Timer 控件和 Label 控件。
首先,在设计窗体时,在窗体上放置一个 Label 控件,并设置其大小和位置。然后,将 Timer 控件拖放到窗体上,并设置其属性,如 Interval,即为跑马灯滚动的时间间隔。
在代码中,可以使用 Timer 控件的 Tick 事件来实现 Label 控件的文本滚动效果。代码示例:
```csharp
private void timer1_Tick(object sender, EventArgs e)
{
string text = label1.Text;
char firstChar = text;
label1.Text = text.Substring(1) + firstChar;
}
```
在 Tick 事件中,首先获取 Label 控件的文本内容,然后将第一个字符取出并添加到字符串末尾,再将新的字符串赋值给 Label 控件的 Text 属性,从而实现文本滚动的效果。
相关问题
用winform 做个跑马灯 ★☆★☆★☆★☆★☆★☆★☆★☆★☆★☆★☆★☆
Winform 是 Windows 程序界面的一种开发框架,可以用于快速构建 Windows 应用程序的图形界面。下面是实现跑马灯的步骤:
1.在 Visual Studio 中新建一个 Windows Forms 应用程序。
2.在窗体上放置一个 Label 控件,设置它的 Text 属性为要显示的文本,如“★☆★☆★☆★☆★☆★☆★☆★☆”。
3.设置 Label 控件的 Dock 属性为 Top,这样它就会沿着窗体的顶部滚动。
4.在窗体的 Load 事件中启动一个计时器,将其 Interval 属性设置为一定的时间间隔(比如100毫秒),并将 Enabled 属性设置为 true。
5.在计时器的 Tick 事件中,每次将 Label 控件的 Text 属性向左移动一定距离(比如5个像素),并判断是否已经移动到窗体的左边界。如果是,则将 Text 属性重新设置为“★☆★☆★☆★☆★☆★☆★☆★☆”并继续滚动。
以下是示例代码:
```
public partial class Form1 : Form
{
private const string marqueeText = "★☆★☆★☆★☆★☆★☆★☆★☆";
private int marqueeOffset = 0;
public Form1()
{
InitializeComponent();
timer1.Interval = 100;
timer1.Enabled = true;
}
private void timer1_Tick(object sender, EventArgs e)
{
marqueeOffset -= 5;
if (marqueeOffset < -label1.Width)
{
marqueeOffset = 0;
label1.Text = marqueeText;
}
label1.Location = new Point(marqueeOffset, 0);
}
}
```
winform写一个跑马灯
以下是一个使用WinForm实现跑马灯效果的示例代码:
```csharp
using System;
using System.Windows.Forms;
namespace WindowsFormsApp
{
public partial class Form1 : Form
{
private Timer timer;
private Label label;
public Form1()
{
InitializeComponent();
InitializeTimer();
InitializeLabel();
}
private void InitializeTimer()
{
timer = new Timer();
timer.Interval = 100; // 设置定时器间隔,单位为毫秒
timer.Tick += Timer_Tick; // 绑定定时器事件
timer.Start(); // 启动定时器
}
private void InitializeLabel()
{
label = new Label();
label.Text = "这是一个跑马灯效果的标签";
label.AutoSize = true;
label.Location = new System.Drawing.Point(10, 10);
Controls.Add(label);
}
private void Timer_Tick(object sender, EventArgs e)
{
// 每次定时器触发时,将标签的文本向左滚动一位
label.Text = label.Text.Substring(1) + label.Text[0];
}
}
}
```
在上述代码中,我们创建了一个`Form1`类,继承自`Form`类,并在构造函数中初始化了定时器和标签。定时器的间隔设置为100毫秒,每次定时器触发时,将标签的文本向左滚动一位。最后,将标签添加到窗体中。
请注意,上述代码仅为示例,实际使用时可能需要根据具体需求进行修改和扩展。
相关推荐
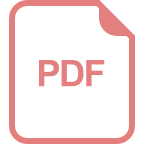
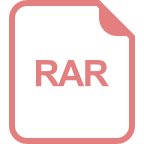
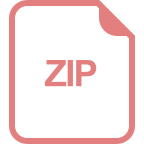












