torchscript **kwargs
时间: 2023-08-13 16:05:17 浏览: 202
在PyTorch中,torchscript是将Python代码转换为静态图形表示的工具,可以提高模型的性能和部署效率。而`**kwargs`是Python语法中的一种特殊参数,用于接收任意数量的关键字参数。在torchscript中,可以使用`**kwargs`来传递额外的参数给torchscript函数或方法。
例如,如果有一个torchscript函数定义如下:
```python
import torch
@torch.jit.script
def my_function(x, **kwargs):
# 可以在函数中使用kwargs中的参数
if 'mode' in kwargs:
mode = kwargs['mode']
# 进行相应操作
# 其他代码逻辑
return x
```
然后,你可以通过传递`**kwargs`来调用该函数并传递额外的关键字参数:
```python
result = my_function(x, mode='train', batch_size=32)
```
在这个例子中,`mode='train'`和`batch_size=32`都是通过`**kwargs`传递给`my_function`的额外关键字参数。
相关问题
How to use **kwargs in torchscript
In PyTorch, **kwargs is a way to pass a variable number of keyword arguments to a function or method. However, **kwargs is not directly supported in TorchScript, the JIT compiler of PyTorch.
To use **kwargs in TorchScript, you can follow these steps:
1. Define a dictionary parameter explicitly in your TorchScript function or method signature to receive the keyword arguments.
2. Pass the keyword arguments as a dictionary when calling the TorchScript function.
Here's an example to demonstrate this:
```python
import torch
from typing import Dict
@torch.jit.script
def my_function(kwargs: Dict[str, int]):
# Access the keyword arguments by their respective keys
if 'arg1' in kwargs:
print(kwargs['arg1'])
if 'arg2' in kwargs:
print(kwargs['arg2'])
# Call the TorchScript function and pass keyword arguments as a dictionary
my_function({'arg1': 10, 'arg2': 20})
```
In the example above, `my_function` takes a dictionary `kwargs` as an argument. Inside the function, you can access the keyword arguments using their respective keys (`arg1` and `arg2` in this case).
Remember to annotate the `kwargs` parameter with type hints to ensure proper type checking by TorchScript.
Traceback (most recent call last): File "D:\Pycharm\PyCharm 2020.2.5\plugins\python\helpers\pydev\pydevd.py", line 1448, in _exec pydev_imports.execfile(file, globals, locals) # execute the script File "D:\Pycharm\PyCharm 2020.2.5\plugins\python\helpers\pydev\_pydev_imps\_pydev_execfile.py", line 18, in execfile exec(compile(contents+"\n", file, 'exec'), glob, loc) File "C:/Users/wz/Desktop/Framework to practice/多尺度Demo/demo.py", line 269, in <module> summary(net, (3, 224, 224)) File "D:\anaconda3\envs\pytorch\lib\site-packages\torchsummary\torchsummary.py", line 72, in summary model(*x) File "D:\anaconda3\envs\pytorch\lib\site-packages\torch\nn\modules\module.py", line 1130, in _call_impl return forward_call(*input, **kwargs) File "C:/Users/wz/Desktop/Framework to practice/多尺度Demo/demo.py", line 228, in forward out = self.MSB3d(out) File "D:\anaconda3\envs\pytorch\lib\site-packages\torch\nn\modules\module.py", line 1148, in _call_impl result = forward_call(*input, **kwargs) File "C:/Users/wz/Desktop/Framework to practice/多尺度Demo/demo.py", line 135, in forward self.branch3x3dbl_3b(branch3x3dbl), File "D:\anaconda3\envs\pytorch\lib\site-packages\torch\nn\modules\module.py", line 1148, in _call_impl result = forward_call(*input, **kwargs) File "C:/Users/wz/Desktop/Framework to practice/多尺度Demo/demo.py", line 42, in forward x = self.conv(x) File "D:\anaconda3\envs\pytorch\lib\site-packages\torch\nn\modules\module.py", line 1148, in _call_impl result = forward_call(*input, **kwargs) File "D:\anaconda3\envs\pytorch\lib\site-packages\torch\nn\modules\conv.py", line 457, in forward return self._conv_forward(input, self.weight, self.bias) File "D:\anaconda3\envs\pytorch\lib\site-packages\torch\nn\modules\conv.py", line 453, in _conv_forward return F.conv2d(input, weight, bias, self.stride, RuntimeError: Given groups=1, weight of size [256, 256, 3, 1], expected input[2, 128, 14, 14] to have 256 channels, but got 128 channels instead
这个错误是由于卷积层的输入通道数不匹配引起的。根据错误信息,期望的输入通道数是256,但实际上却是128。
可能的原因是在定义网络架构时,某个卷积层的输出通道数与下一层的输入通道数不匹配。你可以检查一下代码中的网络定义部分,确保每个卷积层的输出通道数与下一层的输入通道数相等。
另外,你还可以检查一下数据输入部分,确保输入数据的通道数为3(根据代码中的提示,输入的形状应该是[2, 128, 14, 14])。
如果你能提供网络架构和数据输入的代码,我可以更详细地帮助你解决这个问题。
阅读全文
相关推荐

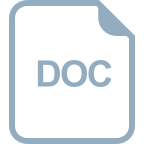
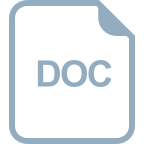











