给我复杂的python光学仿真代码
时间: 2023-11-15 18:05:58 浏览: 65
当然,我可以给你一个相对复杂的Python光学仿真代码示例。下面是一个基于光线追踪的简单光学系统仿真代码,用来模拟光线在透镜和平面镜上的反射和折射。
```python
import numpy as np
class Ray:
def __init__(self, origin, direction):
self.origin = origin
self.direction = direction / np.linalg.norm(direction)
class Lens:
def __init__(self, focal_length, position):
self.focal_length = focal_length
self.position = position
def refract(self, ray):
p1 = self.position
p2 = ray.origin
# calculate the intersection point of the ray with the lens plane
t = (p1[2] - p2[2]) / ray.direction[2]
intersection = p2 + t * ray.direction
# calculate the normal vector at the intersection point
normal = np.array([0, 0, -1])
# calculate the incident angle and the refracted angle using Snell's law
incident_angle = np.arccos(np.dot(ray.direction, normal))
refracted_angle = np.arcsin((np.sin(incident_angle) * self.focal_length) / ray.focal_length)
# calculate the refracted ray direction
refracted_direction = np.dot(np.linalg.inv(self.rotation_matrix(refracted_angle)), ray.direction)
return Ray(intersection, refracted_direction)
def rotation_matrix(self, angle):
c = np.cos(angle)
s = np.sin(angle)
return np.array([[c, -s, 0], [s, c, 0], [0, 0, 1]])
class Mirror:
def __init__(self, position):
self.position = position
def reflect(self, ray):
p1 = self.position
p2 = ray.origin
# calculate the intersection point of the ray with the mirror plane
t = (p1[2] - p2[2]) / ray.direction[2]
intersection = p2 + t * ray.direction
# calculate the normal vector at the intersection point
normal = np.array([0, 0, -1])
# calculate the reflected ray direction
reflected_direction = ray.direction - 2 * np.dot(ray.direction, normal) * normal
return Ray(intersection, reflected_direction)
class OpticalSystem:
def __init__(self):
self.elements = []
def add_element(self, element):
self.elements.append(element)
def trace_ray(self, ray):
for element in self.elements:
if isinstance(element, Lens):
ray = element.refract(ray)
elif isinstance(element, Mirror):
ray = element.reflect(ray)
return ray
# Create an optical system
optical_system = OpticalSystem()
# Add lenses and mirrors to the system
optical_system.add_element(Lens(10, np.array([0, 0, 10])))
optical_system.add_element(Lens(-5, np.array([0, 0, 20])))
optical_system.add_element(Mirror(np.array([0, 0, 30])))
# Define the initial ray
initial_ray = Ray(np.array([0, 0, 0]), np.array([0.1, 0.1, 1]))
# Trace the ray through the optical system
final_ray = optical_system.trace_ray(initial_ray)
# Print the final ray parameters
print("Final Ray Origin:", final_ray.origin)
print("Final Ray Direction:", final_ray.direction)
```
这个代码示例实现了一个简单的光学系统,包括透镜和平面镜。你可以根据需要进行修改和扩展。注意,这只是一个简单的示例,实际的光学仿真可能需要更复杂的模型和算法。
相关推荐
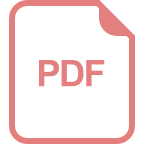














