编写一个java swing 系统,该系统基于java语言连接mysql,该系统要实现基本信息录入、修改、查询等功能: 各种信息的输入,包括项目信息,包括的数据项有:项目编号、项目名称、类型、参加人员、项目经费、实施时间。 项目合作信息的处理。 各种信息的添加、修改、删除。 按照某种条件,查询统计符合条件的各类信息。 对查询、统计的结果打印输出。
时间: 2024-04-13 19:30:02 浏览: 7
你好!对于你的需求,我可以为你提供一个简单的Java Swing系统示例,该系统可以连接MySQL数据库并实现基本信息录入、修改、查询等功能。以下是一个基本的示例代码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.*;
public class InformationSystem extends JFrame {
private JTextField projectNumberField, projectNameField, typeField, participantsField, projectFundingField, implementationTimeField;
private JButton addButton, updateButton, deleteButton, searchButton;
private JTextArea outputArea;
private Connection connection;
private Statement statement;
public InformationSystem() {
setTitle("Information System");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(500, 400);
setLocationRelativeTo(null);
JPanel inputPanel = new JPanel(new GridLayout(7, 2));
inputPanel.add(new JLabel("Project Number:"));
projectNumberField = new JTextField();
inputPanel.add(projectNumberField);
inputPanel.add(new JLabel("Project Name:"));
projectNameField = new JTextField();
inputPanel.add(projectNameField);
inputPanel.add(new JLabel("Type:"));
typeField = new JTextField();
inputPanel.add(typeField);
inputPanel.add(new JLabel("Participants:"));
participantsField = new JTextField();
inputPanel.add(participantsField);
inputPanel.add(new JLabel("Project Funding:"));
projectFundingField = new JTextField();
inputPanel.add(projectFundingField);
inputPanel.add(new JLabel("Implementation Time:"));
implementationTimeField = new JTextField();
inputPanel.add(implementationTimeField);
addButton = new JButton("Add");
addButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
addInformation();
}
});
inputPanel.add(addButton);
updateButton = new JButton("Update");
updateButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
updateInformation();
}
});
inputPanel.add(updateButton);
deleteButton = new JButton("Delete");
deleteButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
deleteInformation();
}
});
inputPanel.add(deleteButton);
searchButton = new JButton("Search");
searchButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
searchInformation();
}
});
inputPanel.add(searchButton);
add(inputPanel, BorderLayout.NORTH);
outputArea = new JTextArea();
JScrollPane scrollPane = new JScrollPane(outputArea);
add(scrollPane, BorderLayout.CENTER);
try {
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/database_name", "username", "password");
statement = connection.createStatement();
} catch (SQLException e) {
e.printStackTrace();
}
}
private void addInformation() {
String projectNumber = projectNumberField.getText();
String projectName = projectNameField.getText();
String type = typeField.getText();
String participants = participantsField.getText();
String projectFunding = projectFundingField.getText();
String implementationTime = implementationTimeField.getText();
String query = "INSERT INTO projects (project_number, project_name, type, participants, project_funding, implementation_time) " +
"VALUES ('" + projectNumber + "', '" + projectName + "', '" + type + "', '" + participants + "', '" + projectFunding + "', '" + implementationTime + "')";
try {
statement.executeUpdate(query);
outputArea.setText("Information added successfully!");
} catch (SQLException e) {
e.printStackTrace();
outputArea.setText("Failed to add information.");
}
}
private void updateInformation() {
// Similar to addInformation(), but with an UPDATE query
// Use WHERE clause to specify the project to update
}
private void deleteInformation() {
// Similar to addInformation(), but with a DELETE query
// Use WHERE clause to specify the project to delete
}
private void searchInformation() {
// Perform SELECT query based on search criteria
// Display the results in the outputArea
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
new InformationSystem().setVisible(true);
}
});
}
}
```
请注意,上面的代码只是一个基本示例,没有包括完整的错误处理、输入验证、连接关闭等。你需要根据实际情况进行修改和完善。
你需要将代码中的 `"database_name"`, `"username"` 和 `"password"` 替换为你自己的MySQL数据库的相关信息。
此外,你还需要创建一个名为 "projects" 的表,该表应包含与项目信息相关的列(例如,project_number、project_name等)。
希望这个示例对你有所帮助!如有任何问题,请随时提问。
相关推荐
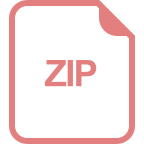
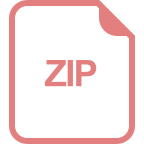
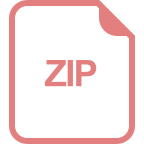














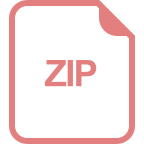