epoll线程池实现高并发服务器c++11
时间: 2024-01-18 22:03:40 浏览: 47
以下是使用epoll和线程池实现高并发服务器的C++11代码示例:
```cpp
#include <iostream>
#include <thread>
#include <vector>
#include <queue>
#include <mutex>
#include <condition_variable>
#include <sys/epoll.h>
#include <unistd.h>
#define MAX_EVENTS 100
#define THREAD_POOL_SIZE 10
std::mutex mtx;
std::condition_variable cv;
std::queue<int> taskQueue;
void workerThread()
{
while (true)
{
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, [] { return !taskQueue.empty(); });
int fd = taskQueue.front();
taskQueue.pop();
// 处理任务,这里可以根据具体需求进行处理
lock.unlock();
// 继续监听其他事件
}
}
int main()
{
// 创建epoll句柄
int epoll_fd = epoll_create(1);
if (epoll_fd == -1)
{
std::cerr << "Failed to create epoll" << std::endl;
return 1;
}
// 创建线程池
std::vector<std::thread> threadPool;
for (int i = 0; i < THREAD_POOL_SIZE; ++i)
{
threadPool.emplace_back(workerThread);
}
// 添加监听事件到epoll句柄
struct epoll_event event;
event.events = EPOLLIN;
event.data.fd = /* 监听的文件描述符 */;
if (epoll_ctl(epoll_fd, EPOLL_CTL_ADD, /* 监听的文件描述符 */, &event) == -1)
{
std::cerr << "Failed to add event to epoll" << std::endl;
return 1;
}
// 开始监听事件
struct epoll_event events[MAX_EVENTS];
while (true)
{
int num_events = epoll_wait(epoll_fd, events, MAX_EVENTS, -1);
if (num_events == -1)
{
std::cerr << "Failed to wait for events" << std::endl;
return 1;
}
for (int i = 0; i < num_events; ++i)
{
if (events[i].events & EPOLLIN)
{
// 处理读事件,将任务添加到任务队列
std::lock_guard<std::mutex> lock(mtx);
taskQueue.push(events[i].data.fd);
cv.notify_one();
}
}
}
// 清理资源
close(epoll_fd);
for (auto& thread : threadPool)
{
thread.join();
}
return 0;
}
```
相关推荐
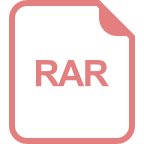
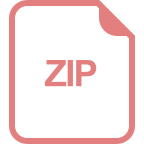














