c++ scanf 怎么读入string类型变量
时间: 2023-08-07 21:10:46 浏览: 208
在C++中,`scanf`函数不能直接读取`std::string`类型的变量,因为`scanf`是C语言的输入函数,无法处理C++的`std::string`。
如果你想读取一个字符串变量,可以使用`std::cin`来实现。`std::cin`是C++中标准输入流对象,用于从控制台读取输入。
以下是使用`std::cin`读取字符串的示例代码:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str;
std::cout << "Enter a string: ";
std::cin >> str;
std::cout << "You entered: " << str << std::endl;
return 0;
}
```
在上述示例中,我们使用`std::cin`来读取用户输入的字符串,并将其存储在名为`str`的`std::string`变量中。然后,我们通过`std::cout`将输入的字符串打印出来。
请注意,使用`std::cin`读取字符串时,它会以空白字符(例如空格、制表符、换行符)为分隔符,只读取第一个单词作为字符串。如果你需要读取整个带有空格的字符串,可以使用`std::getline(std::cin, str)`函数来读取。
相关问题
c++中如何用scanf输入string类型变量
在C++中,`scanf`函数通常用于输入基本数据类型,如整数、浮点数等。但是,如果你想输入字符串(`std::string`),你需要使用稍微复杂一些的方式,因为`scanf`本身并不直接支持`std::string`。
你可以先声明一个空的`std::string`变量,然后使用`getline`函数结合`scanf`来读取一行文本。这里是一个例子:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str;
printf("请输入一个字符串:"); // 提示用户输入
if (scanf("%s", &str) == 1 && !feof(stdin)) { // 使用 %s 和 &str
// 移除末尾的换行符(如果有的话)
str.erase(std::remove(str.begin(), str.end(), '\n'), str.end());
} else {
std::cout << "输入失败!" << std::endl;
}
std::cout << "你输入的字符串是:" << str << std::endl;
return 0;
}
```
在这个例子中,`scanf`的格式 `%s` 会尝试将输入读到`str`的地址,而`!feof(stdin)`是为了检查是否遇到文件结束(EOF),这通常是由于用户按下Enter键而不是输入完整的一行导致的。
注意:`scanf`和`%s`并不是理想的输入字符串的方式,因为它不够安全,容易造成缓冲区溢出。在实际项目中,建议使用`std::getline`配合`cin`来处理字符串输入,这样更安全。
用scanf读入string
scanf函数可以用来读取字符串,但是它无法像直接输入整数那样方便地使用scanf()函数输入string变量。因为string并非是C的原生类型。但是我们可以通过预先分配空间来实现scanf输入string类型的数据。下面是两种方法:
方法一:
```c++
#include <stdio.h>
#include <string>
#include <iostream>
using namespace std;
int main() {
string a;
a.resize(2); // 需要预先分配空间
scanf("%s", &a[0]);
cout << a;
return 0;
}
```
方法二:
```c++
#include<iostream>
#include<string>
using namespace std;
int main() {
string str_scanf;
printf("str_scanf:");
scanf("%s",str_scanf.c_str()); // c方法输入
printf("%s\n", str_scanf.c_str()); // 输出
return 0;
}
```
阅读全文
相关推荐
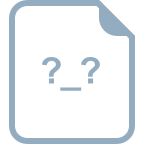
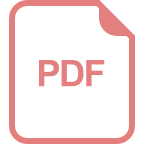
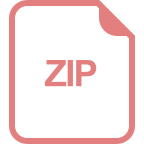
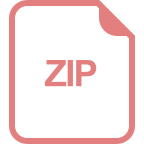
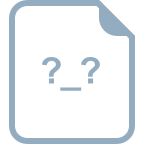
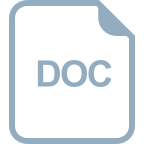
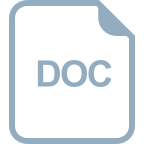
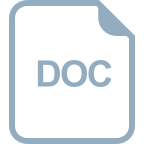
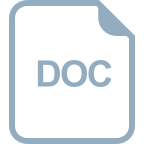
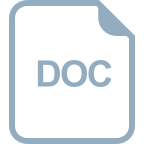
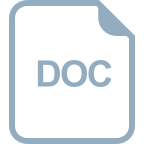
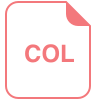



