BitConverter.ToString(arr1).Split('-')
时间: 2024-09-24 11:08:16 浏览: 41
`BitConverter.ToString(arr1).Split('-')` 是 C# 中的一个操作,它主要用于将 `BitConverter.ToHexString(arr1)` 的结果拆分成数组。`BitConverter.ToInt32` 或 `BitConverter.ToUInt32` 等方法通常用于将整数类型的二进制数据转换为十六进制字符串,`ToString` 则进一步将这种十六进制表示形式转换为更易读的形式,如 "FF-EE-DD-CB" 这样的形式,其中 `-` 分隔了每四个十六进制数字。
这里的 `arr1` 应该是一个包含整数字节的数组,`BitConverter.ToString` 会对这个数组进行逐字节的十六进制转换。`Split('-')` 将字符串按照 `-` 分割,返回一个新的字符串数组,每个元素代表原字符串中的一个四字符十六进制块。
举个例子:
```csharp
byte[] arr1 = new byte[] { 0xFF, 0xEE, 0xDD, 0xCB };
string hexString = BitConverter.ToString(arr1);
string[] parts = hexString.Split('-');
// parts 数组现在就是 ["FF", "EE", "DD", "CB"]
```
相关问题
BitConverter.ToString(buffer).Replace("-", "")
`BitConverter.ToString(buffer).Replace("-", "")` 是一个常见的用法,它将字节数组转换为十六进制字符串,并移除了其中的连字符。
例如,假设我们有一个字节数组 `buffer`,其中包含一些数据:
```
byte[] buffer = { 0xAB, 0xCD, 0xEF };
```
我们可以使用 `BitConverter.ToString(buffer).Replace("-", "")` 来将其转换为十六进制字符串,并移除连字符:
```
string hexString = BitConverter.ToString(buffer).Replace("-", "");
```
结果将是一个没有连字符的字符串:"ABCDEF"。这样的字符串在某些情况下很有用,例如在网络通信或数据存储中。
using System; using System.Net; using System.Net.Sockets; using System.Threading.Tasks; using System.Threading; using System.Linq.Expressions; public class TCPClient { private static Socket clientSocket; public static void Main() { // 连接到服务器 Connect("192.168.0.233", 950); try { while (true) ; { // 发送数据 byte[] sendBytes = { 0x01, 0x10, 0x02, 0x58, 0x00, 0x07, 0x0E, 0x00, 0x01, 0x2F, 0x0A, 0x80, 0x01, 0x02, 0x01, 0x10, 0x01, 0x00, 0x02, 0x00, 0x00, 0x5C, 0xA7 }; Send(sendBytes); // 接收数据 byte[] receiveBytes = Receive(); Console.WriteLine("Received message from server: " + BitConverter.ToString(receiveBytes)); Thread.Sleep(500); //等待0.5秒钟 // 发送数据 byte[] sendBytes1 = { 0x01, 0x03, 0x02, 0x58, 0x00, 0x0A, 0x45, 0xA6 }; Send(sendBytes1); // 接收数据 byte[] receiveBytes1 = Receive(); Console.WriteLine("Received message from server: " + BitConverter.ToString(receiveBytes1)); } } catch { clientSocket.Shutdown(SocketShutdown.Both); clientSocket.Close(); } } private static void Connect(string ip, int port) { clientSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp); clientSocket.Connect(new IPEndPoint(IPAddress.Parse(ip), port)); Console.WriteLine("Connected to server."); } private static void Send(byte[] sendBytes) { clientSocket.Send(sendBytes); Console.WriteLine("Sent message to server: " + BitConverter.ToString(sendBytes)); } private static byte[] Receive() { byte[] receiveBytes = new byte[1024]; int length = clientSocket.Receive(receiveBytes); byte[] messageBytes = new byte[length]; Array.Copy(receiveBytes, messageBytes, length); return messageBytes; } private static void Close() { clientSocket.Shutdown(SocketShutdown.Both); clientSocket.Close(); Console.WriteLine("Connection closed."); } }
这段代码是用 C# 编写的 TCP 客户端程序,主要功能是连接到指定的服务器,发送数据并接收数据。程序中使用了 Socket 类来实现网络连接和数据传输。在 Main 函数中,通过 Connect 函数连接到服务器,然后在一个无限循环中不断地发送数据、接收数据,并输出接收到的数据。Send 函数用来发送数据,Receive 函数用来接收数据,Close 函数用来关闭连接。这段代码中使用了 Thread.Sleep 函数来等待一段时间,以便程序能够正常运行。
阅读全文
相关推荐
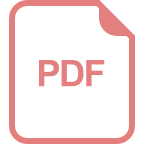
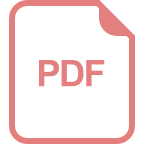
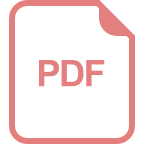











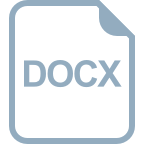