C++将输入的数的偶数位和奇数位分别排序
时间: 2024-12-23 22:19:11 浏览: 4
在C++中,如果你想要将输入数字的偶数位和奇数位分开排序,可以分为两步操作:首先,将数字转换为字符串,并按位分割;然后,对偶数位和奇数位分别进行排序。这里是一个简单的示例,假设我们使用`std::vector<int>`存储每一位:
```cpp
#include <iostream>
#include <sstream>
#include <vector>
#include <algorithm>
// Function to separate even and odd digits
void separate_even_odd(int num, std::vector<int>& evens, std::vector<int>& odds) {
std::stringstream ss;
ss << num; // Convert number to string
std::string str = ss.str();
for (char c : str) {
if (c == '0') {
odds.push_back(atoi(&c)); // Push odd digit (0)
} else {
int digit = c - '1'; // Subtract '0' to get integer value
evens.push_back(digit * 2); // Even digits are doubled (e.g., '1' becomes '2')
odds.push_back(digit); // Odd digits remain unchanged
}
}
}
// Sort the vectors separately
void sort_digits(std::vector<int>& evens, std::vector<int>& odds) {
std::sort(evens.begin(), evens.end());
std::sort(odds.begin(), odds.end());
}
int main() {
int num;
std::cout << "Enter a number: ";
std::cin >> num;
std::vector<int> evens, odds;
separate_even_odd(num, evens, odds);
sort_digits(evens, odds);
// Combine and print back the sorted number
for (const auto& digit : odds) {
num = num / 10 + digit; // Place odd digit back
}
for (const auto& digit : evens) {
num = num * 10 + digit; // Place even digit after odd ones
}
std::cout << "Sorted number with even digits before odd: " << num << std::endl;
return 0;
}
```
在这个程序中,我们首先获取用户输入的整数,然后将其分解成偶数位(通过乘以2表示)和奇数位,接着对它们分别进行排序。最后,我们将排好序的奇数位放在偶数位之前组合回原始数字。
阅读全文
相关推荐
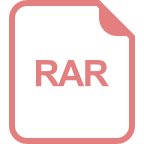
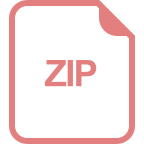
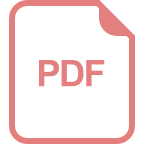















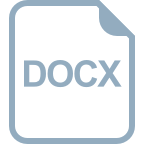