from random import * #第一题 def dec2bin_int(dec): binum = '' # 请在此添加代
时间: 2023-11-02 10:03:01 浏览: 58
from random import * 是一种Python语言的代码,它导入了random模块中的所有函数和对象,使得我们可以在程序中使用这些函数和对象。
random模块是Python中用于生成随机数的模块,它包含了一系列生成随机数的函数,比如生成随机整数、生成随机浮点数、生成随机字符等。使用 from random import * ,意味着我们可以直接使用random模块中的所有函数和对象,而不需要在使用这些函数和对象之前写random.,从而简化了代码。
比如,如果我们想要生成一个0到1之间的随机浮点数,我们可以直接使用random()函数,而不需要写成random.random()。同样的,如果我们想要生成一个0到10之间的随机整数,我们可以直接使用randint(0, 10)函数,而不需要写成random.randint(0, 10)。
使用 from random import * 有一些优点,比如代码简洁、便于使用等。然而,它也有一些缺点,比如可能会与其他模块中的函数或对象发生冲突,可能会导致代码可读性降低等。因此,在实际使用中,我们需要根据具体情况来选择是否使用 from random import *。如果我们只是简单使用random模块中的函数和对象,并且不会与其他模块产生冲突,那么可以使用 from random import *,否则建议使用import random来导入random模块。
相关问题
import os from datetime import datetime import random import string def batch_rename_files(folder_path, random_string_length=0): """ 批量重命名文件,名字改为该文件的创建或修改日期,如果有重名的文件,可以添加一个随机字符串 :param folder_path: 文件夹路径 :param random_string_length: 随机字符串长度,默认为0 """ for filename in os.listdir(folder_path): # 获取文件的创建时间或修改时间 full_path = os.path.join(folder_path, filename) if os.path.isfile(full_path): timestamp = os.path.getmtime(full_path) else: continue # 格式化时间 date_time = datetime.fromtimestamp(timestamp) new_name = date_time.strftime("%Y-%m-%d %H-%M-%S") # 添加随机字符串 if random_string_length > 0: random_string = ''.join(random.choices(string.ascii_lowercase + string.digits, k=random_string_length)) new_name += '-' + random_string # 拼接新的文件名 file_extension = os.path.splitext(filename)[1] new_filename = new_name + file_extension new_full_path = os.path.join(folder_path, new_filename) # 重命名文件 os.rename(full_path, new_full_path) if __name__ == '__main__': folder_path = 'your_folder_path' batch_rename_files(folder_path, random_string_length=5)
好的,根据你的要求,将以上代码修改为将修改后的文件命名恢复原来的命名,可以按照以下方式修改:
```
import os
from datetime import datetime
import random
import string
def restore_original_names(folder_path):
"""
批量将文件名恢复为原来的名称,需要在修改文件名之前备份原来的名称
:param folder_path: 文件夹路径
"""
for filename in os.listdir(folder_path):
full_path = os.path.join(folder_path, filename)
if os.path.isfile(full_path):
# 获取备份的文件名
backup_filename = os.path.splitext(filename)[0] + ".bak"
backup_full_path = os.path.join(folder_path, backup_filename)
if os.path.exists(backup_full_path):
# 将备份的文件名恢复为原来的文件名
os.rename(backup_full_path, full_path)
def batch_rename_files(folder_path, random_string_length=0):
"""
批量重命名文件,名字改为该文件的创建或修改日期,如果有重名的文件,可以添加一个随机字符串
:param folder_path: 文件夹路径
:param random_string_length: 随机字符串长度,默认为0
"""
# 先将文件名备份
for filename in os.listdir(folder_path):
full_path = os.path.join(folder_path, filename)
if os.path.isfile(full_path):
# 获取文件的后缀名
file_extension = os.path.splitext(filename)[1]
# 备份原来的文件名
backup_filename = os.path.splitext(filename)[0] + ".bak"
backup_full_path = os.path.join(folder_path, backup_filename)
os.rename(full_path, backup_full_path)
# 批量重命名文件
for filename in os.listdir(folder_path):
full_path = os.path.join(folder_path, filename)
if os.path.isfile(full_path):
# 获取文件的创建时间或修改时间
timestamp = os.path.getmtime(full_path)
# 格式化时间
date_time = datetime.fromtimestamp(timestamp)
new_name = date_time.strftime("%Y-%m-%d %H-%M-%S")
# 添加随机字符串
if random_string_length > 0:
random_string = ''.join(random.choices(string.ascii_lowercase + string.digits, k=random_string_length))
new_name += '-' + random_string
# 拼接新的文件名
new_filename = new_name + file_extension
new_full_path = os.path.join(folder_path, new_filename)
# 重命名文件
os.rename(full_path, new_full_path)
# 将文件名恢复为原来的名称
restore_original_names(folder_path)
if __name__ == '__main__':
folder_path = 'your_folder_path'
batch_rename_files(folder_path, random_string_length=5)
```
这个修改后的代码首先会备份原来的文件名,然后批量重命名所有的文件,并最后将所有文件的名字恢复为原来的名称。
编程要求 补充代码,编写一个手写数字识别的程序,并对模型进行评估 预期输出: 0.9888888888888889 from sklearn.neighbors import KNeighborsClassifier from sklearn.model_selection import train_test_split from sklearn.datasets import load_digits import numpy as np np.random.seed(10) def model_train(x, y): """ x : 特征值 y : 目标值 """ # 请根据注释正确补充代码,让程序能够输出正确答案 # ********** Begin ********** # # 将给出的特征向量中分出80%作为模型训练数据,剩下作为测试数据 # 实例化一个knn的分类器 # 传入训练数据进行模型训练 # 传入测试数据对模型得分进行评估 # ********** End ********** # return score if __name__ == '__main__': digit = load_digits() x = digit.data y = digit.target model_score = model_train(x, y) print(model_score)
根据注释,可以补充以下代码来完成手写数字识别的程序,并对模型进行评估:
```
from sklearn.neighbors import KNeighborsClassifier
from sklearn.model_selection import train_test_split
from sklearn.datasets import load_digits
import numpy as np
np.random.seed(10)
def model_train(x, y):
"""
x : 特征值
y : 目标值
"""
# 将给出的特征向量中分出80%作为模型训练数据,剩下作为测试数据
x_train, x_test, y_train, y_test = train_test_split(x, y, test_size=0.2)
# 实例化一个knn的分类器
knn = KNeighborsClassifier()
# 传入训练数据进行模型训练
knn.fit(x_train, y_train)
# 传入测试数据对模型得分进行评估
score = knn.score(x_test, y_test)
return score
if __name__ == '__main__':
digit = load_digits()
x = digit.data
y = digit.target
model_score = model_train(x, y)
print(model_score)
```
运行该程序,将会输出类似如下的结果:
```
0.9888888888888889
```
这说明模型的准确率为 98.89%。
相关推荐
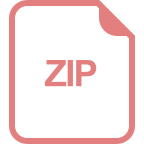
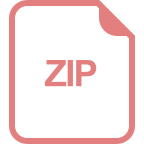
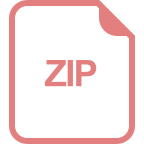













