如何在***企业级应用中利用设计模式实现分层架构,并通过Fowler模式进行数据持久化?请提供具体的实施策略和代码示例。
时间: 2024-11-10 13:31:45 浏览: 15
在企业级应用中,合理利用设计模式对于构建可扩展、可维护和高内聚低耦合的系统至关重要。通过设计模式实现分层架构,可以明确不同组件的职责,使得项目结构清晰,便于团队协作和后期维护。《***设计模式详解:企业级架构与AJAX实践》这本书将引导您理解和掌握这些关键概念,并提供实践中的应用方法。
参考资源链接:[ASP.NET设计模式详解:企业级架构与AJAX实践](https://wenku.csdn.net/doc/72s8zq5xo9?spm=1055.2569.3001.10343)
分层架构通常包括表示层、业务逻辑层和数据访问层。在***应用中,表示层可以使用Web Forms或MVC来构建用户界面,业务逻辑层负责处理业务规则和流程,而数据访问层则负责与数据库交互。实现分层架构的关键是确保层与层之间的通信仅通过定义良好的接口进行,避免直接耦合。
Fowler模式,又称领域驱动设计(Domain-Driven Design,DDD),提供了一套工具和架构模式来处理复杂的业务逻辑。在数据持久化方面,Fowler模式推荐使用Repository模式,该模式作为业务逻辑层与数据访问层之间的中介,负责封装所有对数据的CRUD操作。例如,可以使用NHibernate或Entity Framework作为ORM工具,将对象映射到数据库,并通过Repository模式对外提供操作接口。
具体的实施策略可能包括:
1. 定义数据模型(Entity)和数据传输对象(DTO),区分领域对象和数据传输对象。
2. 创建Repository接口和实现类,使用NHibernate或Entity Framework实现数据的持久化。
3. 在业务逻辑层调用Repository接口完成业务逻辑,并将结果返回给表示层。
4. 使用依赖注入框架,如StructureMap,管理对象的生命周期和依赖关系,提高组件间的解耦。
代码示例(假设使用Entity Framework):
```csharp
// 实体类
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
// Repository接口
public interface IRepository<T> where T : class
{
IEnumerable<T> GetAll();
T GetById(int id);
void Add(T entity);
void Update(T entity);
void Delete(T entity);
}
// Repository实现类
public class ProductRepository : IRepository<Product>
{
private readonly MyDbContext _context;
public ProductRepository(MyDbContext context)
{
_context = context;
}
public IEnumerable<Product> GetAll()
{
return _context.Products.ToList();
}
// 其他方法实现...
}
// 业务逻辑层调用
public class ProductService
{
private readonly IRepository<Product> _productRepository;
public ProductService(IRepository<Product> productRepository)
{
_productRepository = productRepository;
}
public void CreateOrUpdateProduct(Product product)
{
// 检查产品是否存在
var existingProduct = _productRepository.GetById(product.Id);
if (existingProduct != null)
{
_productRepository.Update(product);
}
else
{
_productRepository.Add(product);
}
}
// 其他业务逻辑方法...
}
```
通过这样的架构,您可以有效地利用设计模式来构建稳健的企业级***应用。为了深入理解和实践这些内容,推荐阅读《***设计模式详解:企业级架构与AJAX实践》。该书不仅提供理论知识,还有配套代码示例,有助于您在实际开发中快速应用这些高级设计原则。
参考资源链接:[ASP.NET设计模式详解:企业级架构与AJAX实践](https://wenku.csdn.net/doc/72s8zq5xo9?spm=1055.2569.3001.10343)
阅读全文
相关推荐


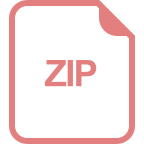
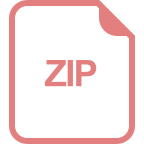
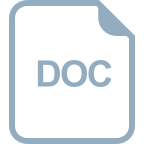
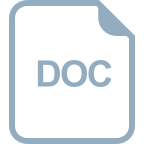
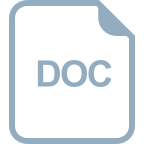
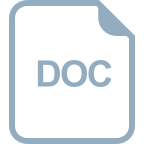
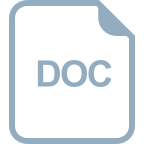
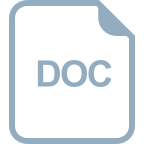
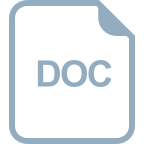
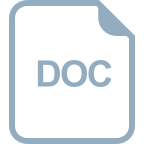
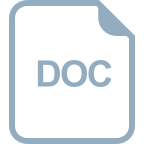
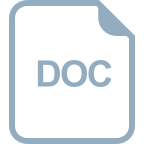
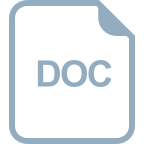
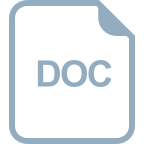
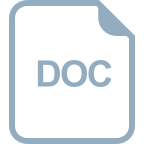
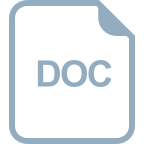
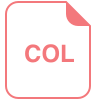
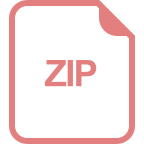