cocos 四叉树怎么写
时间: 2023-09-18 10:03:11 浏览: 33
Cocos 四叉树是一种用于游戏开发中的空间分割数据结构,它可以用于快速检测对象之间的碰撞或者寻找特定区域内的对象。下面是一个简单的实现方法:
首先,我们需要定义一个QuadTree类,该类将代表整个四叉树的数据结构。在其中,我们需要定义树的根节点,树的分割阈值(即每个节点允许的最大对象数量)以及树的边界范围。
接下来,我们需要定义一个QuadNode类,该类代表四叉树中的节点。该节点应包含一个边界范围以及其子节点。如果该节点是叶子节点(即没有子节点),则还应保存该节点范围内的所有对象。
在QuadTree类中,我们需要实现一些基本方法。首先是插入对象的方法。该方法首先检查当前节点是否是叶子节点,如果是,则将对象添加到该节点范围内;如果不是,则根据对象的位置将其插入到当前节点的一个子节点中。如果插入对象后,当前节点的对象数量超过分割阈值,则需要继续分割该节点,并将该节点范围内的对象重新分配到子节点中。
另外,我们还需要实现一个查询给定范围内对象的方法。该方法首先检查查询范围是否与当前节点范围相交,如果不相交,则结束查询;如果相交,则检查当前节点是否是叶子节点,如果是,则返回该节点范围内的所有对象;如果不是,则递归调用该方法来查询子节点。
此外,我们还可以实现一些其他方法,如删除对象、更新对象位置等等,以增强四叉树的功能。
综上所述,我们可以根据上述方式编写一个简单的Cocos四叉树类。当然,这只是一个基本的实现方法,根据实际需求和算法优化,我们还可以对其进行改进。
相关问题
给我一个cocos中使用js实现四叉树碰撞的代码
下面是一个简单的使用 JavaScript 和 Cocos Creator 实现四叉树碰撞检测的代码示例:
```javascript
cc.Class({
extends: cc.Component,
properties: {
// 碰撞检测范围
bounds: cc.Rect,
// 节点是否为叶子节点
isLeaf: false,
// 子节点
children: [],
// 存储的物体列表
objects: [],
// 最大存储数量
maxObjects: 10,
// 最大深度
maxDepth: 4,
// 当前深度
depth: 0
},
onLoad() {
// 初始化四叉树
this.initQuadtree(this.bounds, this.depth);
},
initQuadtree(bounds, depth) {
// 如果当前深度已达到最大深度,或者存储的物体数量已经超过最大存储数量,将该节点标记为叶节点
if (depth >= this.maxDepth || this.objects.length <= this.maxObjects) {
this.isLeaf = true;
return;
}
// 计算四个子矩形的位置和大小
let halfWidth = bounds.width / 2;
let halfHeight = bounds.height / 2;
let leftBounds = cc.rect(bounds.x, bounds.y, halfWidth, bounds.height);
let bottomBounds = cc.rect(bounds.x, bounds.y, bounds.width, halfHeight);
let rightBounds = cc.rect(bounds.x + halfWidth, bounds.y, halfWidth, bounds.height);
let topBounds = cc.rect(bounds.x, bounds.y + halfHeight, bounds.width, halfHeight);
// 创建四个子节点
this.children[0] = new Quadtree(leftBounds, depth + 1);
this.children[1] = new Quadtree(bottomBounds, depth + 1);
this.children[2] = new Quadtree(rightBounds, depth + 1);
this.children[3] = new Quadtree(topBounds, depth + 1);
},
// 将物体添加到四叉树中
insert(object) {
// 如果当前节点不是叶节点,则将物体添加到合适的子节点中
if (!this.isLeaf) {
let index = this.getIndex(object);
this.children[index].insert(object);
return;
}
// 如果当前节点是叶节点,则将物体添加到当前节点存储的物体列表中
this.objects.push(object);
// 如果当前存储的物体数量已经超过最大存储数量,则需要将当前节点分裂为四个子节点
if (this.objects.length > this.maxObjects && this.depth < this.maxDepth) {
for (let i = 0; i < this.objects.length; i++) {
let index = this.getIndex(this.objects[i]);
this.children[index].insert(this.objects[i]);
}
this.objects = [];
this.isLeaf = false;
}
},
// 获取物体所在的子节点索引
getIndex(object) {
let objectBounds = object.getBoundingBox();
let leftBounds = cc.rect(this.bounds.x, this.bounds.y, this.bounds.width / 2, this.bounds.height);
let bottomBounds = cc.rect(this.bounds.x, this.bounds.y, this.bounds.width, this.bounds.height / 2);
let rightBounds = cc.rect(this.bounds.x + this.bounds.width / 2, this.bounds.y, this.bounds.width / 2, this.bounds.height);
let topBounds = cc.rect(this.bounds.x, this.bounds.y + this.bounds.height / 2, this.bounds.width, this.bounds.height / 2);
if (cc.Intersection.rectRect(objectBounds, leftBounds)) {
return 0;
} else if (cc.Intersection.rectRect(objectBounds, bottomBounds)) {
return 1;
} else if (cc.Intersection.rectRect(objectBounds, rightBounds)) {
return 2;
} else if (cc.Intersection.rectRect(objectBounds, topBounds)) {
return 3;
}
return -1;
},
// 获取与物体相交的物体列表
retrieve(object) {
let index = this.getIndex(object);
let objects = this.objects;
if (!this.isLeaf) {
objects = objects.concat(this.children[index].retrieve(object));
}
for (let i = 0; i < objects.length; i++) {
if (object.getBoundingBox().intersects(objects[i].getBoundingBox())) {
result.push(objects[i]);
}
}
return objects;
}
});
```
需要注意的是,这只是一个简单的实现示例,实际使用时可能需要根据具体需求进行优化和修改。
给我一个使用四叉树优化cocos贪吃蛇大作战食物的碰撞检测的js代码
以下是使用四叉树优化碰撞检测的示例代码:
```javascript
// 定义四叉树节点类
class QuadTreeNode {
constructor(x, y, width, height, maxObjects, maxLevels, level) {
this.x = x; // 节点左上角的 x 坐标
this.y = y; // 节点左上角的 y 坐标
this.width = width; // 节点的宽度
this.height = height; // 节点的高度
this.maxObjects = maxObjects; // 节点最多存储的对象数
this.maxLevels = maxLevels; // 四叉树最大深度
this.level = level; // 节点深度
this.objects = []; // 存储在节点中的所有对象
this.nodes = []; // 存储子节点
}
// 分裂节点,将节点分成四个子节点
split() {
const subWidth = this.width / 2;
const subHeight = this.height / 2;
const x = this.x;
const y = this.y;
this.nodes[0] = new QuadTreeNode(x + subWidth, y, subWidth, subHeight, this.maxObjects, this.maxLevels, this.level + 1);
this.nodes[1] = new QuadTreeNode(x, y, subWidth, subHeight, this.maxObjects, this.maxLevels, this.level + 1);
this.nodes[2] = new QuadTreeNode(x, y + subHeight, subWidth, subHeight, this.maxObjects, this.maxLevels, this.level + 1);
this.nodes[3] = new QuadTreeNode(x + subWidth, y + subHeight, subWidth, subHeight, this.maxObjects, this.maxLevels, this.level + 1);
}
// 获取对象所在的子节点
getIndex(rect) {
const verticalMidpoint = this.x + this.width / 2;
const horizontalMidpoint = this.y + this.height / 2;
const topQuadrant = rect.y < horizontalMidpoint && rect.y + rect.height < horizontalMidpoint;
const bottomQuadrant = rect.y > horizontalMidpoint;
let index = -1;
if (rect.x < verticalMidpoint && rect.x + rect.width < verticalMidpoint) {
if (topQuadrant) {
index = 1;
} else if (bottomQuadrant) {
index = 2;
}
} else if (rect.x > verticalMidpoint) {
if (topQuadrant) {
index = 0;
} else if (bottomQuadrant) {
index = 3;
}
}
return index;
}
// 插入对象到四叉树中
insert(rect) {
if (this.nodes.length) {
const index = this.getIndex(rect);
if (index !== -1) {
this.nodes[index].insert(rect);
return;
}
}
this.objects.push(rect);
if (this.objects.length > this.maxObjects && this.level < this.maxLevels) {
if (!this.nodes.length) {
this.split();
}
let i = 0;
while (i < this.objects.length) {
const index = this.getIndex(this.objects[i]);
if (index !== -1) {
this.nodes[index].insert(this.objects.splice(i, 1)[0]);
} else {
i++;
}
}
}
}
// 获取所有与指定对象碰撞的对象
retrieve(rect) {
const index = this.getIndex(rect);
let foundObjects = this.objects;
if (this.nodes.length) {
if (index !== -1) {
foundObjects = foundObjects.concat(this.nodes[index].retrieve(rect));
} else {
for (let i = 0; i < this.nodes.length; i++) {
foundObjects = foundObjects.concat(this.nodes[i].retrieve(rect));
}
}
}
return foundObjects;
}
}
// 定义游戏场景类
class GameScene {
constructor() {
this.snake = null; // 贪吃蛇
this.foods = []; // 食物
this.quadTree = new QuadTreeNode(0, 0, 960, 640, 10, 5, 0); // 四叉树
}
// 初始化游戏场景
init() {
// 创建贪吃蛇和食物
this.snake = new Snake();
for (let i = 0; i < 10; i++) {
const food = new Food();
this.foods.push(food);
this.quadTree.insert(food.rect);
}
}
// 更新游戏场景
update(dt) {
// 移动贪吃蛇
this.snake.move(dt);
// 检测贪吃蛇与食物的碰撞
const snakeRect = this.snake.getRect();
const collidedFoods = this.quadTree.retrieve(snakeRect);
for (let i = 0; i < collidedFoods.length; i++) {
const food = collidedFoods[i];
if (this.snake.checkCollision(food.rect)) {
// 贪吃蛇吃到了食物
this.snake.eat(food);
// 从场景中移除食物
this.foods.splice(this.foods.indexOf(food), 1);
this.quadTree.objects.splice(this.quadTree.objects.indexOf(food.rect), 1);
// 创建新的食物并加入场景
const newFood = new Food();
this.foods.push(newFood);
this.quadTree.insert(newFood.rect);
}
}
}
// 渲染游戏场景
render() {
// 渲染贪吃蛇和食物
this.snake.render();
for (let i = 0; i < this.foods.length; i++) {
this.foods[i].render();
}
}
}
// 定义食物类
class Food {
constructor() {
this.rect = new Rect(Math.floor(Math.random() * 960), Math.floor(Math.random() * 640), 10, 10);
}
render() {
// 渲染食物
}
}
// 定义矩形类
class Rect {
constructor(x, y, width, height) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
}
// 定义贪吃蛇类
class Snake {
constructor() {
this.body = [new Rect(100, 100, 10, 10), new Rect(90, 100, 10, 10), new Rect(80, 100, 10, 10)];
this.direction = 'right';
}
// 移动贪吃蛇
move(dt) {
// 根据方向移动贪吃蛇
}
// 检测贪吃蛇与其他对象的碰撞
checkCollision(rect) {
// 检测贪吃蛇头部是否与指定矩形相交
}
// 贪吃蛇吃到食物
eat(food) {
// 在贪吃蛇尾部添加一个新的矩形
}
// 获取贪吃蛇的矩形
getRect() {
// 返回贪吃蛇头部的矩形
}
render() {
// 渲染贪吃蛇
}
}
// 创建游戏场景对象并初始化
const gameScene = new GameScene();
gameScene.init();
// 游戏循环
function gameLoop(dt) {
// 更新游戏场景
gameScene.update(dt);
// 渲染游戏场景
gameScene.render();
// 继续下一帧循环
requestAnimationFrame(gameLoop);
}
// 开始游戏循环
requestAnimationFrame(gameLoop);
```
以上代码仅为示例,实际使用时需要根据项目需求进行修改。
相关推荐
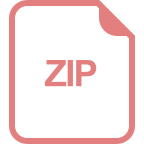
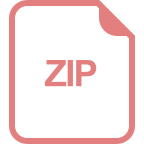












