帮我用python写一段使用socket实现服务端数据客户端接受并使用十六进制加密的代码
时间: 2024-10-09 11:01:41 浏览: 22
当然可以,以下是使用Python的socket模块实现简单的服务端和服务端通信,其中包含了客户端的数据接收以及对数据的十六进制加密的示例。我们将假设使用了一个简单的加密函数`hex_encrypt`:
```python
import socket
from Crypto.Cipher import AES
# 加密函数,这里仅作演示,实际应用应使用更安全的库如pycryptodome
def hex_encrypt(data, key):
cipher = AES.new(key.encode('utf-8'), AES.MODE_EAX)
ciphertext, tag = cipher.encrypt_and_digest(data.encode('utf-8'))
return cipher.nonce + tag + ciphertext.hex()
# 服务器端(接收客户端连接)
def server():
host = 'localhost'
port = 12345
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind((host, port))
s.listen(1)
conn, addr = s.accept()
print(f"Connected by {addr[0]}:{addr[1]}")
# 接收客户端发送的数据
while True:
data = conn.recv(1024).decode('utf-8')
if not data:
break
# 对接收到的数据进行加密
encrypted_data = hex_encrypt(data, "your_secret_key") # 替换为你的密钥
conn.sendall(encrypted_data)
conn.close()
s.close()
# 客户端(向服务器发送数据)
def client():
host = 'localhost'
port = 12345
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect((host, port))
# 发送数据前先连接上服务器
message = "Hello Server!" # 您想发送的消息
encrypted_message = hex_encrypt(message, "your_secret_key") # 使用相同的密钥
s.sendall(encrypted_message)
received_data = s.recv(1024).decode('utf-8')
print(f"Received from server: {received_data}")
s.close()
# 运行示例
if __name__ == "__main__":
server_thread = threading.Thread(target=server)
client_thread = threading.Thread(target=client)
server_thread.start()
client_thread.start()
```
在这个例子中,我们创建了两个线程,一个是服务器,一个是客户端。客户端发送消息到服务器,服务器接收到后加密后再返回给客户端。注意,为了加密,这里使用了`Crypto.Cipher.AES`,这是一个模拟的例子,实际生产环境应使用更安全的加密库。
阅读全文
相关推荐
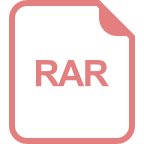
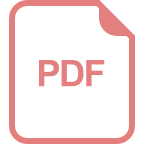
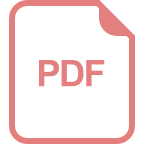
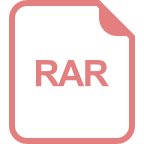
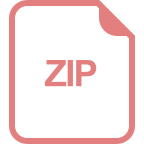
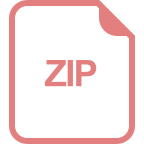
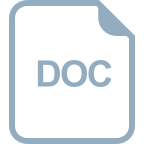


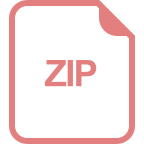
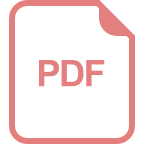
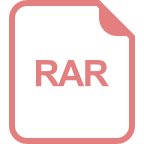
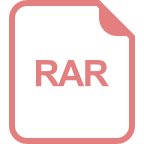
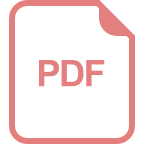
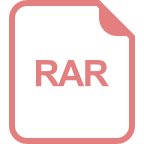
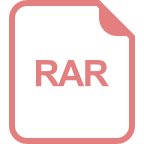
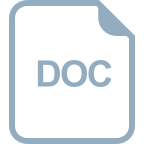

