c++怎么在TXT中提取数字
时间: 2024-10-09 13:01:37 浏览: 25
在C++中,如果你想从TXT文件中提取数字,可以使用标准库中的`std::getline()`配合`std::istringstream`或者`std::regex`来进行解析。下面是一个基本的例子,使用`std::istringstream`来分割字符串:
```cpp
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
std::vector<double> extract_numbers_from_text(const std::string& filename) {
std::ifstream file(filename);
if (!file.is_open()) {
throw std::runtime_error("Failed to open the file");
}
std::vector<double> numbers;
std::string line;
while (std::getline(file, line)) {
double number;
std::istringstream iss(line);
if (!(iss >> number)) { // 如果无法读取到一个数字,说明可能是非数字字符
std::cerr << "Invalid or non-number detected in line: " << line << '\n';
} else {
numbers.push_back(number);
}
}
return numbers;
}
int main() {
try {
const auto numbers = extract_numbers_from_text("your_file.txt");
for (double num : numbers) {
std::cout << num << '\n';
}
} catch (const std::exception &e) {
std::cerr << e.what() << '\n';
}
return 0;
}
```
这个代码片段会在每行中尝试读取一个数字。如果遇到非数字字符,它会捕获异常并打印错误消息。结果会保存在一个`double`类型的`vector`中。
如果你需要更复杂的模式匹配,例如科学计数法或正则表达式,你可以考虑使用`std::regex`库。然而,对于简单的数字提取,上述方法已经足够了。
阅读全文
相关推荐
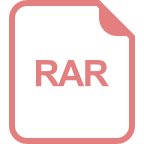
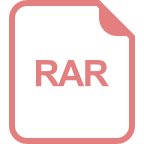
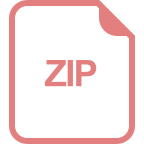
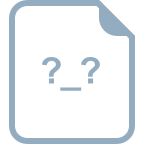
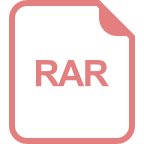
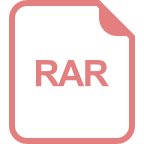
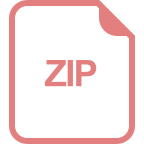
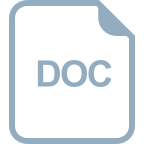
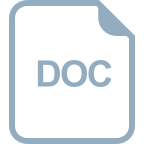







