利用bert的训练加权文件做测试代码
时间: 2024-04-11 21:26:13 浏览: 12
以下是一个示例代码,展示了如何利用BERT的训练加权文件进行测试。请注意,这只是一个基本的代码框架,具体实现可能因你使用的工具库和任务类型而有所不同。
```python
import torch
from transformers import BertTokenizer, BertForSequenceClassification
# 加载预训练的BERT模型和tokenizer
model = BertForSequenceClassification.from_pretrained('bert-base-uncased')
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
# 加载训练加权文件
test_file = 'test.txt' # 替换为你的测试数据文件路径
with open(test_file, 'r', encoding='utf-8') as f:
lines = f.readlines()
# 处理测试数据
input_ids = []
attention_masks = []
labels = []
for line in lines:
parts = line.strip().split('\t')
text = parts[0]
label = int(parts[1])
# 使用tokenizer对文本进行编码
encoded_dict = tokenizer.encode_plus(
text,
add_special_tokens=True,
max_length=128,
pad_to_max_length=True,
return_attention_mask=True,
return_tensors='pt'
)
input_ids.append(encoded_dict['input_ids'])
attention_masks.append(encoded_dict['attention_mask'])
labels.append(label)
# 将数据转换为PyTorch张量
input_ids = torch.cat(input_ids, dim=0)
attention_masks = torch.cat(attention_masks, dim=0)
labels = torch.tensor(labels)
# 设置模型为评估模式
model.eval()
# 前向传播并预测结果
with torch.no_grad():
outputs = model(input_ids, attention_mask=attention_masks)
# 对于分类任务,获取预测结果
predictions = torch.argmax(outputs.logits, dim=1)
# 计算准确率
accuracy = (predictions == labels).float().mean().item()
print("Accuracy:", accuracy)
```
在这个示例代码中,我们使用了Hugging Face的`transformers`库,它提供了方便的BERT模型和tokenizer的加载和使用。你需要根据你的具体情况替换文件路径、模型名称等信息。
请注意,以上代码仅适用于文本分类任务,对于其他类型的任务,你需要根据具体需求修改模型、数据处理和评估部分的代码。另外,还可以根据需要添加更多的评估指标和后处理步骤。
相关推荐
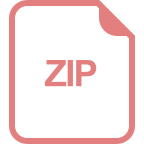
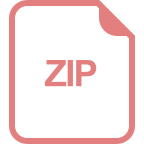














