在C++编程中,如何分别统计一个字符串中的大写字母数量、小写字母数量以及数字的个数呢?
时间: 2024-10-20 22:03:30 浏览: 30
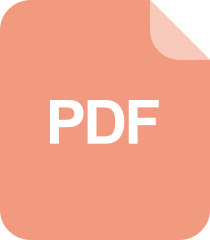
C++统计中英文大小写字母、数字、空格及其他字符个数的方法

在C++中,你可以通过遍历字符串并使用字符分类来进行计数。这里是一个简单的例子:
```cpp
#include <iostream>
#include <cctype> // 包含ASCII函数库,用于转换字符
int countUpperCase(const std::string& str) {
int count = 0;
for (char c : str) {
if(isupper(c)) { // 判断是否为大写字母
count++;
}
}
return count;
}
int countLowerCase(const std::string& str) {
int count = 0;
for (char c : str) {
if(islower(c)) { // 判断是否为小写字母
count++;
}
}
return count;
}
int countDigits(const std::string& str) {
int count = 0;
for (char c : str) {
if(isdigit(c)) { // 判断是否为数字
count++;
}
}
return count;
}
int main() {
std::string inputStr;
std::cout << "Enter a string: ";
std::getline(std::cin, inputStr);
int uppercaseCount = countUpperCase(inputStr);
int lowercaseCount = countLowerCase(inputStr);
int digitCount = countDigits(inputStr);
std::cout << "Uppercase letters: " << uppercaseCount << "\n";
std::cout << "Lowercase letters: " << lowercaseCount << "\n";
std::cout << "Digits: " << digitCount << "\n";
return 0;
}
```
在这个程序中,`isupper`, `islower`, 和 `isdigit` 是从 `<cctype>` 库获取的ASCII函数,用于检查字符是否分别为大写字母、小写字母和数字。
阅读全文
相关推荐
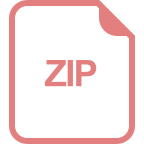
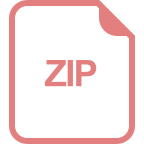















