c++ 反向查找字符第n次
时间: 2024-10-25 09:13:57 浏览: 18
在C++中,如果你想找到一个字符串中某个字符最后一次出现的位置(即第n次出现),可以使用`std::string::find_last_of()`函数配合循环来实现。这个函数会在指定的字符集中查找最后一个匹配的字符,并返回其位置。如果你想要找到特定字符的第n次出现,你需要先找到所有出现的位置,然后从后向前数到第n次。
下面是一个简单的示例:
```cpp
#include <iostream>
#include <string>
int findNthOccurrence(const std::string& str, char target, int n) {
size_t pos = str.find(target);
if (pos == std::string::npos || n <= 0) {
return -1; // 如果未找到目标字符或者n小于等于0,返回-1表示找不到
}
for (size_t i = 1; i < n; ++i) {
pos = str.find(target, pos + 1); // 从上次找到的位置后开始查找
if (pos == std::string::npos) {
break; // 如果未找到更多次,提前退出
}
}
return static_cast<int>(pos); // 返回字符最后一次出现的位置,注意转换成整型
}
int main() {
std::string str = "Hello, World! Hello";
char target = 'l';
int n = 2; // 查找第二次出现
int nthPos = findNthOccurrence(str, target, n);
if (nthPos != -1) {
std::cout << "Character '" << target << "' is found at position " << nthPos << " for the " << n << "th time." << std::endl;
} else {
std::cout << "Character not found that many times." << std::endl;
}
return 0;
}
```
在这个例子中,我们首先检查是否找到了目标字符,如果没有或n小于等于0,则返回-1。接着,在循环里不断查找,直到找到第n次。
阅读全文
相关推荐
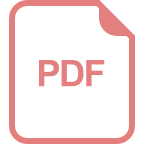
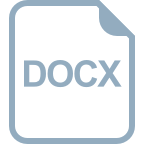
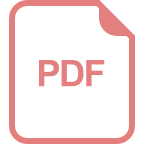



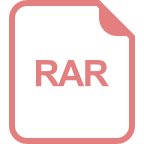
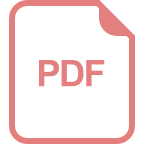
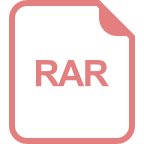
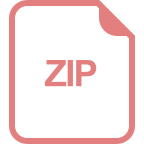
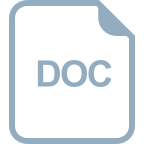
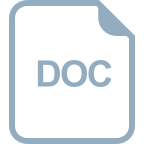
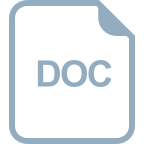
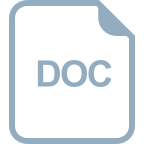
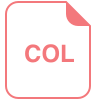
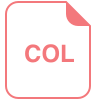
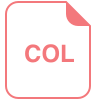
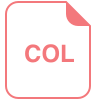
