python实现keras库中的神经网络优化器衰减
时间: 2024-11-24 21:40:38 浏览: 20
在Keras库中,你可以使用`tf.keras.optimizers`模块来创建并应用各种神经网络优化器,其中包含多种类型的衰减策略。例如,如果你想在Adam优化器中添加学习率衰减,可以利用`LearningRateSchedule`接口。以下是如何在Python中实现这一点的一个例子:
```python
import tensorflow as tf
from tensorflow.keras.optimizers.schedules import LearningRateSchedule, ExponentialDecay
class CustomLRSchedule(LearningRateSchedule):
def __init__(self, initial_learning_rate, decay_steps, decay_rate=0.96):
super(CustomLRSchedule, self).__init__()
self.initial_learning_rate = initial_learning_rate
self.decay_steps = decay_steps
self.decay_rate = decay_rate
def call(self, step):
return self.initial_learning_rate * (self.decay_rate ** tf.math.floor(step / self.decay_steps))
# 使用自定义学习率衰减的Adam优化器
initial_learning_rate = 0.001
decay_steps = 1000
custom_decay = CustomLRSchedule(initial_learning_rate, decay_steps)
optimizer = tf.keras.optimizers.Adam(learning_rate=custom_decay)
# 在训练循环中,学习率会随着训练步骤自动衰减
for step in range(total_steps):
with tf.GradientTape() as tape:
# 计算梯度
gradients = tape.gradient(loss, model.trainable_variables)
optimizer.apply_gradients(zip(gradients, model.trainable_variables))
```
在这个例子中,`CustomLRSchedule`继承自`LearningRateSchedule`,并在`call`方法中计算每个时间步的衰减后的学习率。然后,在创建Adam优化器时,我们将这个衰减函数传递给了`learning_rate`参数。
阅读全文
相关推荐
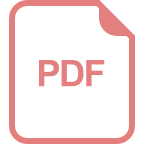
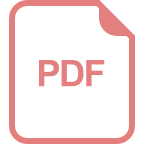
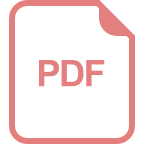
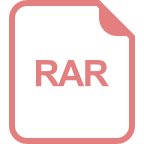
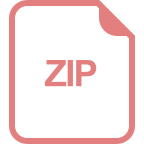
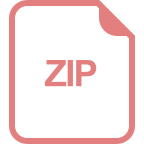
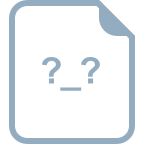
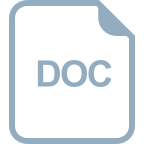
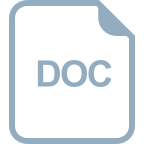
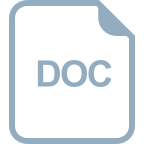
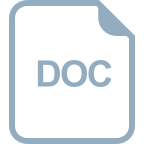
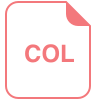
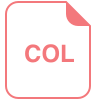
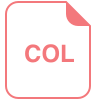
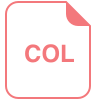
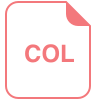
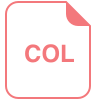

