编写程序实现 PCB 的链接存储结构,完成以下功能。 (1)创建带头结点的 PCB 链表; (2)创建就绪状态的 PCB 并加入链表; (3)删除指定的 PCB; (4)显示链表中的 PCB 信息。
时间: 2024-10-16 13:11:58 浏览: 36
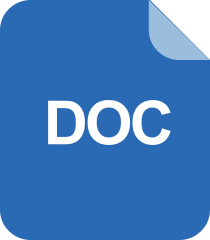
操作系统实验报告- 进程状态转换及其PCB的变化

在编写程序以实现 PCB(进程控制块)的链接存储结构时,你需要创建一种数据结构来管理每个进程的状态,并支持添加、删除和查看操作。以下是基本步骤:
1. **创建带头节点的 PCB 链表**:
- 定义一个 PCB 结构体,包含进程ID、CPU状态(如运行、等待等)、优先级、队列指针等必要字段。
- 创建一个 PCB 链表,可以使用单链表或双向链表,其中头结点是一个特殊的 PCB,用于表示链表的起始。
```python
class PCB:
def __init__(self, pid, status, priority):
self.pid = pid
self.status = status
self.priority = priority
self.next = None # 如果是单链表
self.prev = None # 如果是双向链表
head = PCB(0, "UNUSED", 0) # 初始化头结点
```
2. **创建就绪状态的 PCB 并加入链表**:
- 创建新的 PCB 实例,设置其状态为“就绪”,然后将其添加到链表的适当位置,通常是在就绪队列头部(如果按优先级排序)。
```python
def create_new_pcbs():
new_pcb = PCB(new_pid, "READY", priority)
if head.next is None:
head.next = new_pcb
new_pcb.prev = head
else:
last_pcb = find_last_node(head)
last_pcb.next = new_pcb
new_pcb.prev = last_pcb
```
3. **删除指定的 PCB**:
- 通过遍历链表找到要删除的 PCB,然后更新前后节点的引用,如果是头结点,则需要特殊处理。
```python
def delete_pcb(pid):
current = head
while current and current.pid != pid:
current = current.next
if current:
if current == head:
head = current.next
if head:
head.prev = None
else:
current.prev.next = current.next
if current.next:
current.next.prev = current.prev
del current
```
4. **显示链表中的 PCB 信息**:
- 使用循环遍历链表并打印每个 PCB 的相关信息。
```python
def display_pcbs():
current = head
while current:
print(f"PID: {current.pid}, Status: {current.status}, Priority: {current.priority}")
current = current.next
```
阅读全文
相关推荐
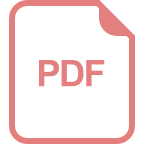
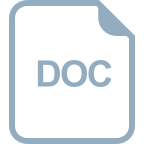



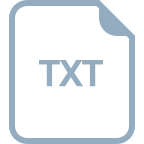
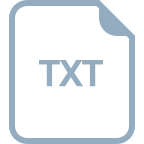
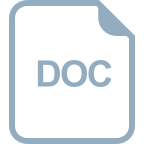
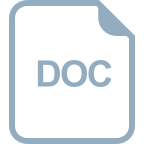
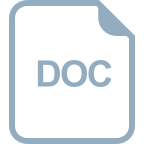
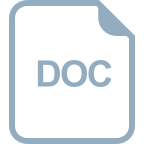
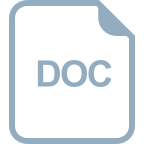
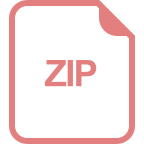
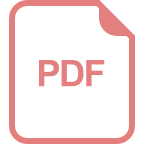
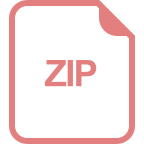
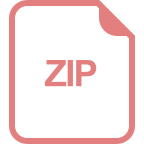
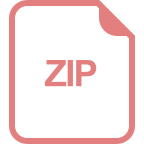