定义一个顺序表Sqlist,其数据元素是整数,要求数据元素不仅有唯一的前驱和后继,还要有序,如升序,参考下面的实验指导修改插入算法,或者再写一个插入算法,实现有序插入,用随机产生的10个100以内的整数,建立一个数据元素升序的顺序表,输出结果 c语言
时间: 2024-10-14 20:13:11 浏览: 33
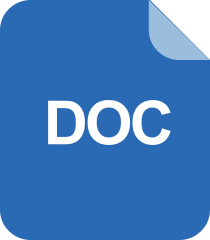
数据结构实验报告顺序表的创建遍历及有序合并操作优质资料.doc
在C语言中,我们可以定义一个名为`Sqlist`的顺序表结构体,用于存储整数并保持有序(升序)。这里我们假设已有一个`insert_sorted`函数实现了有序插入。首先,我们需要定义结构体以及相关的头文件。
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// 结构体定义
typedef struct Sqlist {
int data; // 整数值
struct Sqlist* next; // 指向下一个元素的指针
} Sqlist;
// 插入有序的函数
void insert_sorted(Sqlist** head, int value) {
if (*head == NULL || value <= (*head)->data) {
Sqlist* newNode = (Sqlist*)malloc(sizeof(Sqlist));
newNode->data = value;
newNode->next = *head;
*head = newNode;
} else {
Sqlist* current = *head;
while (current->next != NULL && current->next->data < value) {
current = current->next;
}
if (current->next != NULL) {
Sqlist* newNode = (Sqlist*)malloc(sizeof(Sqlist));
newNode->data = value;
newNode->next = current->next;
current->next = newNode;
} else {
Sqlist* newNode = (Sqlist*)malloc(sizeof(Sqlist));
newNode->data = value;
newNode->next = NULL;
current->next = newNode;
}
}
}
int main() {
srand(time(0)); // 设置随机种子
Sqlist* head = NULL; // 初始链表为空
for (int i = 0; i < 10; i++) {
int randomValue = rand() % 100 + 1; // 生成100以内的随机数
printf("Adding %d...\n", randomValue);
insert_sorted(&head, randomValue);
}
// 输出结果
Sqlist* current = head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
return 0;
}
```
在这个程序中,我们创建了一个`Sqlist`结构体,定义了插入有序的`insert_sorted`函数,并通过循环生成10个随机数插入到列表中。最后,遍历整个列表并打印出结果。
阅读全文
相关推荐
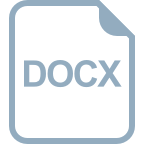
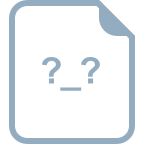















