vue3 antd 父组件调用子组件modal
时间: 2023-11-17 09:05:19 浏览: 167
在Vue3中,可以通过`ref`和`teleport`来实现父组件调用子组件的modal。
首先,在子组件中,需要使用`teleport`将modal渲染到body中,这样可以避免modal被父组件的样式影响。代码如下:
```html
<template>
<teleport to="body">
<div class="modal">
<!-- modal内容 -->
</div>
</teleport>
</template>
```
然后,在父组件中,需要使用`ref`来获取子组件的实例,并通过调用子组件的方法来打开或关闭modal。代码如下:
```html
<template>
<div>
<button @click="openModal">打开modal</button>
<ChildComponent ref="childComponentRef" />
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent,
},
methods: {
openModal() {
this.$refs.childComponentRef.openModal();
},
},
};
</script>
```
在子组件中,需要定义一个`openModal`方法来打开modal,并通过`emits`来向父组件发送事件。代码如下:
```html
<template>
<teleport to="body">
<div class="modal" v-if="visible">
<!-- modal内容 -->
</div>
</teleport>
</template>
<script>
import { defineEmits } from 'vue';
export default {
emits: ['update:visible'],
data() {
return {
visible: false,
};
},
methods: {
openModal() {
this.visible = true;
this.$emit('update:visible', true);
},
closeModal() {
this.visible = false;
this.$emit('update:visible', false);
},
},
};
</script>
```
在父组件中,需要通过`v-model`来监听子组件的`visible`属性,并在子组件的`update:visible`事件触发时更新`visible`属性。代码如下:
```html
<template>
<div>
<button @click="visible = true">打开modal</button>
<ChildComponent v-model:visible="visible" />
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent,
},
data() {
return {
visible: false,
};
},
};
</script>
```
这样,就可以实现父组件调用子组件的modal了。
阅读全文
相关推荐
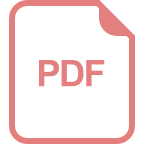
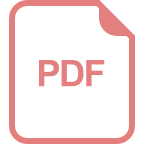

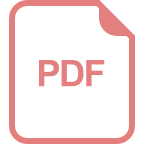
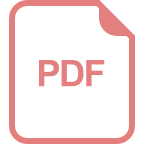
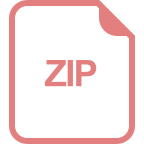
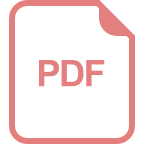
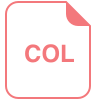
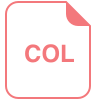
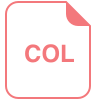
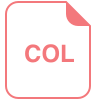
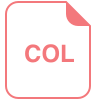





