torch.sum示例
时间: 2023-08-03 11:02:18 浏览: 49
`torch.sum()` 函数用于计算张量(tensor)沿着指定维度的元素之和。它的使用方式如下:
```python
torch.sum(input, dim=None, keepdim=False, dtype=None)
```
其中:
- `input`:需要计算元素之和的输入张量。
- `dim`:可选,指定计算元素之和的维度。如果不指定,则计算所有元素之和。
- `keepdim`:可选,是否保持输出张量的维度与输入张量相同,默认为 False。
- `dtype`:可选,指定输出张量的数据类型,默认与输入张量相同。
下面是一个示例:
```python
import torch
x = torch.tensor([[1, 2, 3], [4, 5, 6]])
y = torch.sum(x, dim=1)
print(y)
```
输出结果为:
```
tensor([ 6, 15])
```
在这个例子中,我们创建了一个形状为 2 行 3 列的张量 `x`。我们使用 `torch.sum()` 函数计算了 `x` 沿着第二个维度(即列)的元素之和,得到了一个形状为 2 的新张量 `y`。其中,第一行的元素之和为 6,第二行的元素之和为 15。
相关问题
torch.sum函数介绍
torch.sum函数是PyTorch库中的一个张量操作函数,用于计算张量中所有元素的总和。它可以在给定的维度上对张量进行求和,也可以计算整个张量的总和。
函数签名如下:
```python
torch.sum(input, dim=None, keepdim=False, dtype=None)
```
参数说明:
- `input`:输入的张量。
- `dim`:指定在哪个维度上进行求和操作。如果不指定,则对整个张量进行求和。
- `keepdim`:指定是否保持输出张量的维度与输入张量相同。默认为False。
- `dtype`:指定输出张量的数据类型。如果不指定,则使用输入张量的数据类型。
返回值:
- 返回一个新的张量,其中包含了输入张量在指定维度上的求和结果。
示例:
```python
import torch
x = torch.tensor([[1, 2, 3], [4, 5, 6]])
# 对整个张量进行求和
sum_all = torch.sum(x)
print(sum_all) # 输出tensor(21)
# 按列求和
sum_dim0 = torch.sum(x, dim=0)
print(sum_dim0) # 输出tensor([5, 7, 9])
# 按行求和
sum_dim1 = torch.sum(x, dim=1)
print(sum_dim1) # 输出tensor([ 6, 15])
```
这是torch.sum函数的基本介绍,它在处理张量数据时非常常用。
实现一个Linear层(torch.nn.Linear)功能,分别基于torch.nn.Module和torch.autograd.Function
好的,下面分别给出基于`torch.nn.Module`和`torch.autograd.Function`的实现示例。
基于`torch.nn.Module`的实现示例:
```python
import torch
import torch.nn as nn
class LinearLayer(nn.Module):
def __init__(self, input_size, output_size):
super(LinearLayer, self).__init__()
self.weight = nn.Parameter(torch.Tensor(output_size, input_size))
self.bias = nn.Parameter(torch.Tensor(output_size))
self.reset_parameters()
def reset_parameters(self):
nn.init.kaiming_uniform_(self.weight, a=math.sqrt(5))
if self.bias is not None:
fan_in, _ = nn.init._calculate_fan_in_and_fan_out(self.weight)
bound = 1 / math.sqrt(fan_in)
nn.init.uniform_(self.bias, -bound, bound)
def forward(self, input):
return torch.matmul(input, self.weight.t()) + self.bias
```
基于`torch.autograd.Function`的实现示例:
```python
import torch
from torch.autograd import Function
class LinearFunction(Function):
@staticmethod
def forward(ctx, input, weight, bias=None):
ctx.save_for_backward(input, weight, bias)
output = torch.matmul(input, weight.t())
if bias is not None:
output += bias.unsqueeze(0).expand_as(output)
return output
@staticmethod
def backward(ctx, grad_output):
input, weight, bias = ctx.saved_tensors
grad_input = grad_weight = grad_bias = None
if ctx.needs_input_grad[0]:
grad_input = torch.matmul(grad_output, weight)
if ctx.needs_input_grad[1]:
grad_weight = torch.matmul(grad_output.t(), input)
if bias is not None and ctx.needs_input_grad[2]:
grad_bias = grad_output.sum(0)
return grad_input, grad_weight, grad_bias
class LinearLayer(Function):
@staticmethod
def forward(ctx, input, weight, bias=None):
ctx.save_for_backward(input, weight, bias)
output = torch.matmul(input, weight.t())
if bias is not None:
output += bias.unsqueeze(0).expand_as(output)
return output
@staticmethod
def backward(ctx, grad_output):
input, weight, bias = ctx.saved_tensors
grad_input = grad_weight = grad_bias = None
if ctx.needs_input_grad[0]:
grad_input = torch.matmul(grad_output, weight)
if ctx.needs_input_grad[1]:
grad_weight = torch.matmul(grad_output.t(), input)
if bias is not None and ctx.needs_input_grad[2]:
grad_bias = grad_output.sum(0)
return grad_input, grad_weight, grad_bias
```
这两个示例分别基于`torch.nn.Module`和`torch.autograd.Function`实现了一个Linear层。你可以根据需要选择其中一种实现方式。希望对你有所帮助!
相关推荐
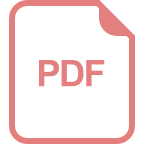
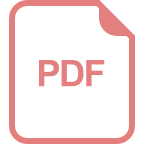












