flink 乱序数据排序
时间: 2023-08-14 22:06:54 浏览: 134
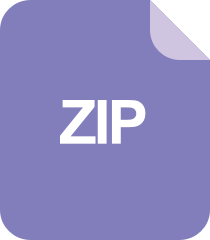
对各种数据进行排序展示
在 Flink 中,要对乱序数据进行排序,可以使用 Flink 的 `KeyedStream` 和 `ProcessFunction` 来实现。下面是一个示例代码:
```java
import org.apache.flink.api.common.functions.MapFunction;
import org.apache.flink.api.java.tuple.Tuple2;
import org.apache.flink.streaming.api.datastream.DataStream;
import org.apache.flink.streaming.api.environment.StreamExecutionEnvironment;
import org.apache.flink.streaming.api.functions.KeyedProcessFunction;
import org.apache.flink.util.Collector;
public class FlinkSortExample {
public static void main(String[] args) throws Exception {
StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
DataStream<Tuple2<String, Integer>> input = env.fromElements(
new Tuple2<>("key1", 5),
new Tuple2<>("key2", 3),
new Tuple2<>("key1", 2),
new Tuple2<>("key2", 1)
);
DataStream<Tuple2<String, Integer>> sortedOutput = input
.keyBy(0) // 根据指定的 key 进行分组
.process(new SortProcessFunction());
sortedOutput.print();
env.execute("Flink Sort Example");
}
public static class SortProcessFunction extends KeyedProcessFunction<String, Tuple2<String, Integer>, Tuple2<String, Integer>> {
@Override
public void processElement(Tuple2<String, Integer> value, Context ctx, Collector<Tuple2<String, Integer>> out) throws Exception {
// 将接收到的数据缓存起来
ctx.timerService().registerEventTimeTimer(ctx.timestamp());
ctx.getBroadcastState().put(ctx.timestamp(), value);
}
@Override
public void onTimer(long timestamp, OnTimerContext ctx, Collector<Tuple2<String, Integer>> out) throws Exception {
// 当定时器触发时,遍历缓存的数据并输出
for (Tuple2<String, Integer> value : ctx.getBroadcastState().values()) {
out.collect(value);
}
// 清空缓存
ctx.getBroadcastState().clear();
}
}
}
```
在上面的示例中,我们使用 `keyBy` 方法对输入流进行分组,然后使用 `process` 方法将数据存储在状态中,并在定时器触发时输出排序后的数据。在实际应用中,你可能需要根据具体的需求进行调整和优化。
阅读全文
相关推荐
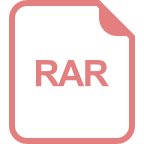
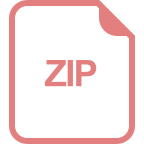


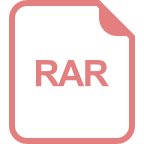
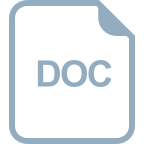
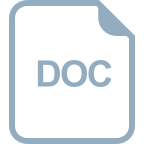
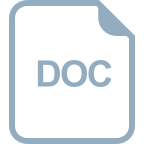
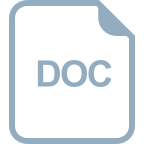
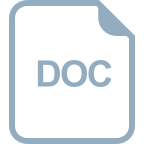
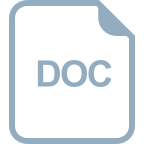
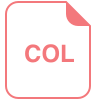
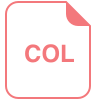
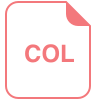
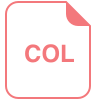
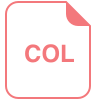
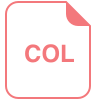
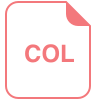