用C语言写凯撒密码,输入输出也写
时间: 2024-10-10 18:17:18 浏览: 51
凯撒密码是一种简单的替换加密技术,通过将字母表中的每个字符向右移动固定的位置来进行加密。以下是使用C语言编写的一个基本的凯撒密码程序,包括输入和输出:
```c
#include <stdio.h>
#include <string.h>
// 定义加密函数,偏移量设为3(经典案例)
char caesar_cipher(char c, int shift) {
if (c >= 'A' && c <= 'Z') { // 大写字母处理
return ((c - 'A' + shift) % 26) + 'A';
} else if (c >= 'a' && c <= 'z') { // 小写字母处理
return ((c - 'a' + shift) % 26) + 'a';
}
return c; // 其他字符保持不变
}
int main() {
char plaintext[100], ciphertext[100];
int shift;
printf("请输入明文:\n");
fgets(plaintext, sizeof(plaintext), stdin);
plaintext[strcspn(plaintext, "\n")] = '\0'; // 清除换行符
printf("请输入偏移量(例如1表示 Caesar Cipher):\n");
scanf("%d", &shift);
for (int i = 0; plaintext[i]; ++i) {
ciphertext[i] = caesar_cipher(plaintext[i], shift);
}
ciphertext[strcspn(ciphertext, "\n")] = '\0'; // 再次清除换行符
printf("加密后的密文是:\n");
printf("%s\n", ciphertext);
return 0;
}
```
在这个程序中,用户首先输入明文,然后选择一个偏移量(这里默认是3,可以修改)。接下来,`caesar_cipher` 函数会处理每个字符并按照选定的偏移量进行加密。最后,程序打印出加密后的密文。
阅读全文
相关推荐
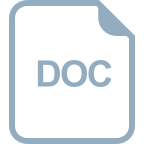
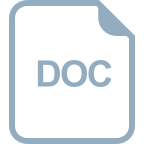
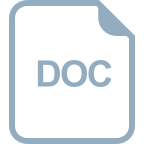


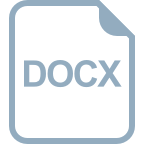
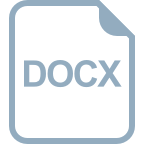
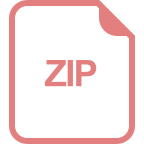
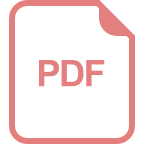
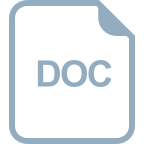
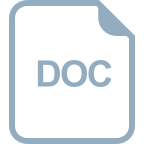
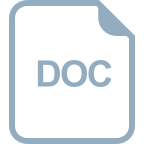
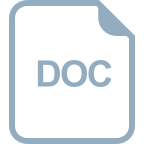
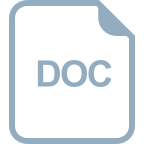
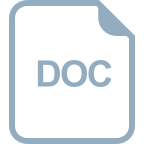



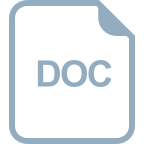