Pun的第一个基于熵的阈值分割用python实现
时间: 2024-09-28 12:03:38 浏览: 40
Pun是一个基于信息熵(Entropy)的图像分割算法,也称为最小熵法或Shannon Entropy Method。它的核心思想是寻找一种划分方式,使得整个图像的信息不确定性(由熵度量)降到最低。在Python中实现这样一个算法,你需要遵循以下步骤:
1. 导入必要的库:首先,你需要`skimage`库来进行图像处理,以及`numpy`用于数学计算。
```python
import numpy as np
from skimage import measure, io, filters
```
2. 加载和预处理图像:读取图像并转换为灰度,以便于后续处理。
```python
img = io.imread('your_image.png', as_gray=True)
```
3. 计算像素概率分布:对于每个像素,计算其属于各个可能区域的概率。
```python
probabilities = filters.rank_histogram(img, n_bins=256)
```
4. 计算信息熵:使用信息熵公式(对每一个概率p,熵H(p)=-p*log2(p),对于0<p<1),求得整个图像的熵。
```python
def entropy(probabilities):
ent = -np.sum(probabilities * np.log2(probabilities))
return ent
entropy_value = entropy(probabilities)
```
5. 设定阈值:选择一个合适的阈值,通常是通过比较当前熵值与一个预先设定的阈值来决定是否继续分割。
```python
threshold_entropy = some_predefined_threshold
if entropy_value > threshold_entropy:
# 继续分割
else:
# 达到阈值,停止分割
```
6. 分割过程:根据熵值递减原则,从高熵区域开始,逐步合并临近区域直到达到阈值为止。可以使用`measure.label`函数来标记分割后的区域。
```python
labels = measure.label(entropy_map < threshold_entropy)
```
7. 结果可视化:最后,可以用不同颜色表示不同的区域。
```python
segmented_img = measure.regionprops(labels, img)
io.imshow(segmented_img)
```
阅读全文
相关推荐


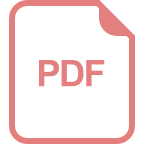















