vue+springboot文件上传和下载
时间: 2024-12-26 10:19:14 浏览: 7
### 使用 Vue 和 Spring Boot 实现文件上传和下载
#### 文件上传部分
为了实现文件上传的功能,前端使用 Vue.js 来构建用户界面,并通过 HTTP 请求将文件发送给后端服务器。而后端则采用 Spring Boot 接收这些请求并将文件存储起来。
##### 前端代码示例(Vue)
在 Vue 中可以通过 `axios` 库发起 POST 请求来提交表单数据以及文件流:
```javascript
import axios from 'axios';
export default {
methods: {
async submitFile() {
const formData = new FormData();
formData.append('file', this.selectedFile);
try {
await axios.post('/api/upload', formData, {
headers: {
'Content-Type': 'multipart/form-data'
}
});
console.log('Upload successful');
} catch (error) {
console.error('There was an error uploading the file!', error);
}
},
handleFileSelect(event){
this.selectedFile = event.target.files[0];
}
}
}
```
此段脚本定义了一个名为 `submitFile()` 的方法用于处理文件的选择与上传操作[^1]。
##### 后端代码示例(Spring Boot)
对于接收文件的部分,在控制器类中声明一个映射路径 `/upload` 并设置其支持 POST 方法。当有新的文件被上传时会触发该接口下的函数执行相应的业务逻辑:
```java
@RestController
@RequestMapping("/api")
public class FileUploadController {
@PostMapping("/upload")
public ResponseEntity<String> uploadFile(@RequestParam("file") MultipartFile file) throws IOException {
if(file.isEmpty()){
return ResponseEntity.status(HttpStatus.BAD_REQUEST).body("Please select a file to upload.");
}
// Save uploaded file on server...
Path path = Paths.get(UPLOADED_FOLDER + file.getOriginalFilename());
Files.copy(file.getInputStream(), path, StandardCopyOption.REPLACE_EXISTING);
return ResponseEntity.ok("You successfully uploaded '" + file.getOriginalFilename() + "'");
}
}
```
这段 Java 代码片段展示了如何创建 RESTful API 来接受来自客户端的多部件形式的数据包,并将其保存至指定位置上[^2]。
#### 文件下载部分
同样地,要让应用程序能够响应用户的下载请求也需要前后两端共同协作完成这项工作。
##### 前端代码示例(Vue)
为了让用户可以从浏览器获取远程资源,可以在组件内部编写如下所示的方法调用:
```javascript
async downloadFile(filename) {
try{
let response = await fetch(`/api/download/${filename}`);
const blob = await response.blob();
var link=document.createElement('a');
link.href=URL.createObjectURL(blob);
link.download = filename;
document.body.appendChild(link);
link.click();
URL.revokeObjectURL(link.href);
document.body.removeChild(link);
}catch(error){
console.error('Error during downloading:', error.message);
}
},
```
上述 JavaScript 函数负责向特定地址发出 GET 请求以取得目标二进制对象,之后再利用 HTML5 提供的新特性自动弹出另存对话框让用户选择保存地点[^4]。
##### 后端代码示例(Spring Boot)
最后一步是在服务层准备好可供访问的目标实体及其元信息以便于返回给调用者:
```java
@GetMapping("/download/{fileName:.+}")
@ResponseBody
public FileSystemResource getFile(
@PathVariable String fileName,
HttpServletResponse response) throws Exception {
Path filePath = Paths.get(DOWNLOAD_PATH + "/" + fileName);
if (!Files.exists(filePath)) {
throw new FileNotFoundException(fileName + " not found on server");
}
response.setContentType(MediaType.APPLICATION_OCTET_STREAM_VALUE);
response.setHeader(HttpHeaders.CONTENT_DISPOSITION,"attachment; filename=\"" + URLEncoder.encode(fileName,StandardCharsets.UTF_8.toString())+"\"");
return new FileSystemResource(filePath.toFile());
}
```
这个处理器用来匹配所有形如 `/download/*` 的 URI 模式,并尝试读取对应的磁盘上的实际文件作为回应的一部分传回给客户机。
阅读全文
相关推荐
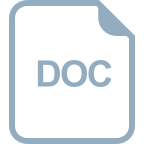
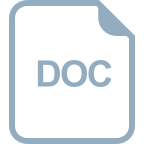
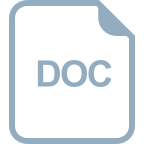
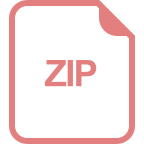
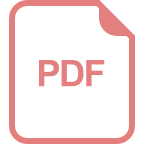



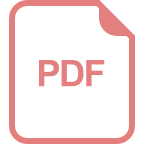
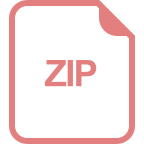
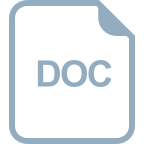
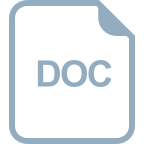






