ElementUI+vue+springboot图片上传
时间: 2023-09-18 13:05:46 浏览: 73
首先,你需要使用 ElementUI 中的 Upload 组件来实现图片上传。具体步骤如下:
1. 在 Vue 组件中引入 ElementUI 的 Upload 组件:
```
<template>
<el-upload
class="upload-demo"
action="/api/upload"
:on-success="handleSuccess"
:before-upload="beforeUpload"
:limit="1"
:file-list="fileList"
:auto-upload="false">
<el-button slot="trigger" size="small" type="primary">选取文件</el-button>
<el-button style="margin-left: 10px;" size="small" type="success" @click="submitUpload">上传到服务器</el-button>
<div slot="tip" class="el-upload__tip">只能上传jpg/png文件,且不超过500kb</div>
</el-upload>
</template>
```
2. 在 Vue 组件中定义上传文件的处理函数 handleSuccess:
```
<script>
export default {
data() {
return {
fileList: []
};
},
methods: {
handleSuccess(response, file, fileList) {
console.log(response);
this.fileList = fileList;
},
beforeUpload(file) {
const isJPG = file.type === "image/jpeg" || file.type === "image/png";
const isLt500K = file.size / 1024 < 500;
if (!isJPG || !isLt500K) {
this.$message.error("上传图片只能是 JPG/PNG 格式,且不超过 500KB");
}
return isJPG && isLt500K;
},
submitUpload() {
this.$refs.upload.submit();
}
}
};
</script>
```
3. 在后端 SpringBoot 中编写上传文件的 API:
```
@RestController
@RequestMapping("/api")
public class FileUploadController {
@PostMapping("/upload")
public ResponseEntity<?> uploadFile(@RequestParam("file") MultipartFile file) {
try {
// 存储文件
byte[] bytes = file.getBytes();
Path path = Paths.get("uploads/" + file.getOriginalFilename());
Files.write(path, bytes);
// 返回成功信息
return ResponseEntity.ok("File uploaded successfully");
} catch (IOException e) {
e.printStackTrace();
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
}
}
}
```
4. 在前端 Vue 中设置上传文件的 API 地址为后端 SpringBoot 中编写的 API 地址:
```
<el-upload
class="upload-demo"
action="/api/upload"
:on-success="handleSuccess"
:before-upload="beforeUpload"
:limit="1"
:file-list="fileList"
:auto-upload="false">
...
</el-upload>
```
这样,你就可以在前端使用 ElementUI+Vue 实现图片上传,并在后端使用 SpringBoot 接收上传的图片文件了。
相关推荐
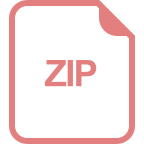
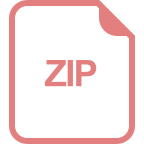
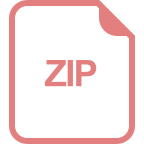














