选取一个合适的数据结构存储数据,设计一学生成绩表,能对数据进行插入、 删除、绕计总分、按总分从大到小排序、查找等。
时间: 2024-12-22 22:12:47 浏览: 11
为了设计一个学生成绩表,我们可以选择使用关联数组(如Python中的字典或JavaScript的Map,以及许多其他编程语言中的类似数据结构),这种数据结构能够方便地存储每个学生的信息,包括姓名作为键(key)和成绩(值)。下面是一个简单的示例:
```python
class StudentScoreTable:
def __init__(self):
self.students = {} # 使用字典存储,键为学生名,值为成绩列表
# 插入成绩
def add_score(self, student_name, score):
if student_name not in self.students:
self.students[student_name] = []
self.students[student_name].append(score)
# 删除成绩
def delete_score(self, student_name, score):
if student_name in self.students and score in self.students[student_name]:
self.students[student_name].remove(score)
# 计算总分
def calculate_total_score(self, student_name):
if student_name in self.students:
return sum(self.students[student_name])
else:
return 0
# 按总分从大到小排序
def sort_by_total_score(self):
sorted_students = {k: v for k, v in sorted(self.students.items(), key=lambda item: sum(item[1]), reverse=True)}
return sorted_students
# 查找学生总分
def find_total_score(self, student_name):
return self.calculate_total_score(student_name)
# 使用示例
table = StudentScoreTable()
table.add_score("张三", 95)
table.add_score("李四", 88)
table.sort_by_total_score() # {"张三": [95], "李四": [88]}
print(table.find_total_score("张三")) # 输出:95
```
阅读全文
相关推荐












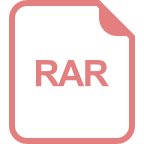





