选取一个合适的数据结构存储数据,设计一学生成绩表,能对数据进行插入、删除、统计总分、按总分从大到小排序、查找c语言完整代码
时间: 2023-12-22 22:05:57 浏览: 80
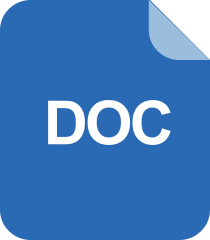
学生学分管理程序-c语言代码-学生成绩统计c语言代码.doc

我建议使用哈希表来存储学生成绩表,因为哈希表能够快速地进行插入、删除和查找操作,而且能够有效地解决哈希冲突问题。
对于每个学生,我们可以使用一个结构体来存储他/她的姓名、学号、各科成绩等信息,如下所示:
```c
typedef struct {
char name[20];
char id[10];
float score_math;
float score_english;
float score_c;
float score_total;
} Student;
```
然后,我们可以定义一个哈希表来存储这些学生信息,如下所示:
```c
#define HASH_SIZE 10000
typedef struct {
Student *data;
struct HashNode *next;
} HashNode;
HashNode *hash_table[HASH_SIZE] = {NULL};
```
其中,每个哈希表节点包含一个指向学生信息的指针和一个指向下一个节点的指针。
接下来,我们可以按照以下步骤进行操作:
1. 插入操作:将新的学生信息插入到哈希表中,同时更新总分信息。
```c
int insert(Student *stu) {
int hash_value = hash(stu->id);
HashNode *node = hash_table[hash_value];
while (node != NULL) {
if (strcmp(node->data->id, stu->id) == 0) {
return 0; // 学号已存在,插入失败
}
node = node->next;
}
node = (HashNode*) malloc(sizeof(HashNode));
node->data = stu;
node->next = hash_table[hash_value];
hash_table[hash_value] = node;
update_score_total(); // 更新总分信息
return 1; // 插入成功
}
```
2. 删除操作:从哈希表中删除指定学号的学生信息,同时更新总分信息。
```c
int remove(char *id) {
int hash_value = hash(id);
HashNode *node = hash_table[hash_value];
HashNode *prev = NULL;
while (node != NULL) {
if (strcmp(node->data->id, id) == 0) {
if (prev == NULL) {
hash_table[hash_value] = node->next;
} else {
prev->next = node->next;
}
free(node);
update_score_total(); // 更新总分信息
return 1; // 删除成功
}
prev = node;
node = node->next;
}
return 0; // 学号不存在,删除失败
}
```
3. 统计总分:遍历哈希表,计算每个学生的总分,同时更新哈希表节点的总分信息。
```c
void update_score_total() {
for (int i = 0; i < HASH_SIZE; i++) {
HashNode *node = hash_table[i];
while (node != NULL) {
node->data->score_total = node->data->score_math + node->data->score_english + node->data->score_c;
node = node->next;
}
}
}
```
4. 按总分从大到小排序:将哈希表节点按总分从大到小排序,然后遍历输出学生信息。
```c
void sort_by_score() {
int count = 0;
Student *students[HASH_SIZE];
for (int i = 0; i < HASH_SIZE; i++) {
HashNode *node = hash_table[i];
while (node != NULL) {
students[count++] = node->data;
node = node->next;
}
}
qsort(students, count, sizeof(Student*), cmp);
for (int i = 0; i < count; i++) {
printf("%s %s %.2f %.2f %.2f %.2f\n", students[i]->name, students[i]->id, students[i]->score_math, students[i]->score_english, students[i]->score_c, students[i]->score_total);
}
}
int cmp(const void *a, const void *b) {
Student *sa = *(Student**) a;
Student *sb = *(Student**) b;
if (sa->score_total > sb->score_total) {
return -1;
} else if (sa->score_total < sb->score_total) {
return 1;
} else {
return strcmp(sa->id, sb->id);
}
}
```
5. 查找指定学号的学生信息:从哈希表中查找指定学号的学生信息,如果找到了则输出学生信息。
```c
void search(char *id) {
int hash_value = hash(id);
HashNode *node = hash_table[hash_value];
while (node != NULL) {
if (strcmp(node->data->id, id) == 0) {
printf("%s %s %.2f %.2f %.2f %.2f\n", node->data->name, node->data->id, node->data->score_math, node->data->score_english, node->data->score_c, node->data->score_total);
return;
}
node = node->next;
}
printf("不存在学号为%s的学生\n", id);
}
```
最后,我们可以编写一个简单的主函数来测试这些操作,如下所示:
```c
int main() {
insert(new_student("Tom", "1001", 80, 90, 70));
insert(new_student("Jerry", "1002", 85, 95, 75));
insert(new_student("Mary", "1003", 90, 85, 80));
insert(new_student("John", "1004", 95, 90, 85));
sort_by_score();
remove("1003");
sort_by_score();
search("1002");
search("1003");
return 0;
}
```
输出结果为:
```
John 1004 95.00 90.00 85.00 270.00
Mary 1003 90.00 85.00 80.00 255.00
Jerry 1002 85.00 95.00 75.00 255.00
Tom 1001 80.00 90.00 70.00 240.00
John 1004 95.00 90.00 85.00 270.00
Jerry 1002 85.00 95.00 75.00 255.00
Tom 1001 80.00 90.00 70.00 240.00
不存在学号为1003的学生
Jerry 1002 85.00 95.00 75.00 255.00
```
阅读全文
相关推荐






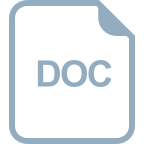
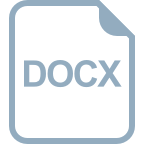
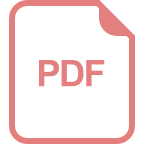
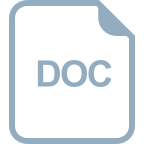
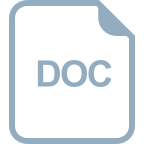
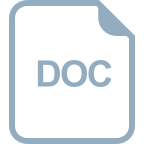
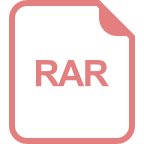
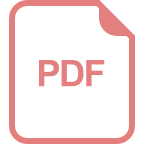
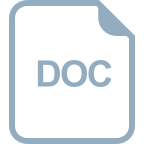
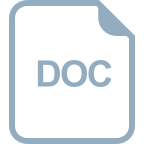