Python制图多条折线
时间: 2024-09-26 14:09:00 浏览: 28
在Python中,我们可以使用matplotlib库来创建多条折线图。以下是一个基本的例子,展示了如何绘制两条不同的折线:
```python
import matplotlib.pyplot as plt
# 创建数据点
x = [0, 1, 2, 3, 4, 5]
line1_data = [1, 4, 9, 16, 25, 36] # 第一条线的数据
line2_data = [0, 1, 4, 9, 16, 25] # 第二条线的数据
# 创建图形并设置标题
plt.figure(figsize=(10, 6))
plt.title('Multiple Line Plots')
# 绘制两条折线
plt.plot(x, line1_data, label='Line 1', color='red') # 红色折线
plt.plot(x, line2_data, label='Line 2', color='blue') # 蓝色折线
# 添加图例
plt.legend()
# 显示图形
plt.show()
```
在这个例子中,`plot()`函数用于创建线条,`label=`参数用于给每条线添加标签,方便后续添加图例。最后通过`legend()`显示图例,并用`show()`来显示整个图形。
相关问题
数据库读取数据Python制图多条折线
在Python中,我们可以使用一些强大的库如pandas和matplotlib来处理数据库读取、数据分析以及生成多条折线图。以下是一个简单的流程:
1. **数据库读取**:
- 首先,你需要安装必要的库,例如`pymysql`或`sqlite3`(如果你的数据存储在SQLite),以及`pandas`来连接和操作数据库。
- 使用`pandas.read_sql_query()`或`read_sql_table()`函数从MySQL或SQLite数据库中加载数据到DataFrame中。
```python
import pandas as pd
# 连接到SQLite数据库
if 'sqlite' in your_database_url:
df = pd.read_sql_query('SELECT * FROM table_name', con=your_connection)
# 或者连接到MySQL
elif 'mysql' in your_database_url:
query = "SELECT column1, column2 FROM your_table"
df = pd.read_sql(query, con=mysql_engine)
```
2. **数据处理**:
对读取的数据进行清洗、整理和计算,以便创建折线图。
```python
df['date_column'] = pd.to_datetime(df['date_column']) # 如果需要日期作为x轴
df.set_index('date_column', inplace=True) # 设置时间序列作为索引
# 创建数据框组,如果有多个系列需要在同一张图上展示
lines_data = {name: df[name] for name in ['series1', 'series2']}
```
3. **制图**:
使用`matplotlib`库中的`pyplot`模块绘制折线图,可以同时画出多条折线。
```python
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
for series_name, data in lines_data.items():
ax.plot(data.index, data.values, label=series_name)
ax.legend() # 显示图例
ax.set_xlabel('Date') # x轴标签
ax.set_ylabel('Value') # y轴标签
plt.title('Multiple Line Plots from Database') # 图表标题
plt.show()
```
python绘制多条折线图
好的,那么您想知道如何用Python绘制多条折线图是吗?可以使用Python的matplotlib库来完成这个任务。以下是一个基本的示例代码:
```python
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y1 = [1, 3, 2, 4, 5]
y2 = [2, 1, 5, 3, 4]
plt.plot(x, y1)
plt.plot(x, y2)
plt.xlabel('x-axis')
plt.ylabel('y-axis')
plt.title('Multiple Line Graph')
plt.show()
```
这个例子中,我们创建了两个y轴数组y1和y2,它们都关于相同的x轴数组x进行绘图。通过在plt.plot()函数中分别传递这两个y轴数组,我们可以同时绘制两条折线。最后,使用plt.xlabel()、plt.ylabel()和plt.title()函数添加标签和标题,再使用plt.show()函数显示图形。
阅读全文
相关推荐
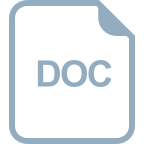
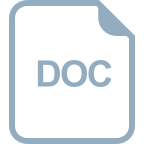
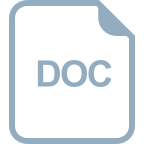
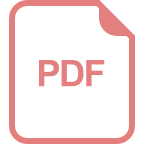




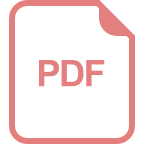
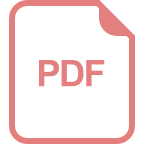
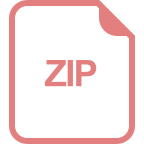





